Using URLs as a Webhook
Last updated 17/07/2024
Overview
Creating a URL that uses a process allows you to fully customize a public URL to be processed with code. Your code will need to be attached to the Do Run event on your process. The Do Run event is a server (backend) event and when the data has been submitted from your external site, your URL created in Five will be used to receive the data and insert it into your database, effectively acting as a webhook from an external site.
To achieve this, you will need to perform the following steps:
- Create a function, in the function you will need to use Five's parametersproperty.
- Create a process and attach your function to the Do Run event.
- Create a URL and reference your process.
URL and Data Flow
When the URL has been sent a request with the
When you have completed what you need to do, simply return
Using a Curl Command
Curl is a command-line tool that allows you to do HTTP requests from the shell. Curl stands for 'Client URL'. You can invoke it from your preferred terminal.
The default behavior for curl is to invoke a
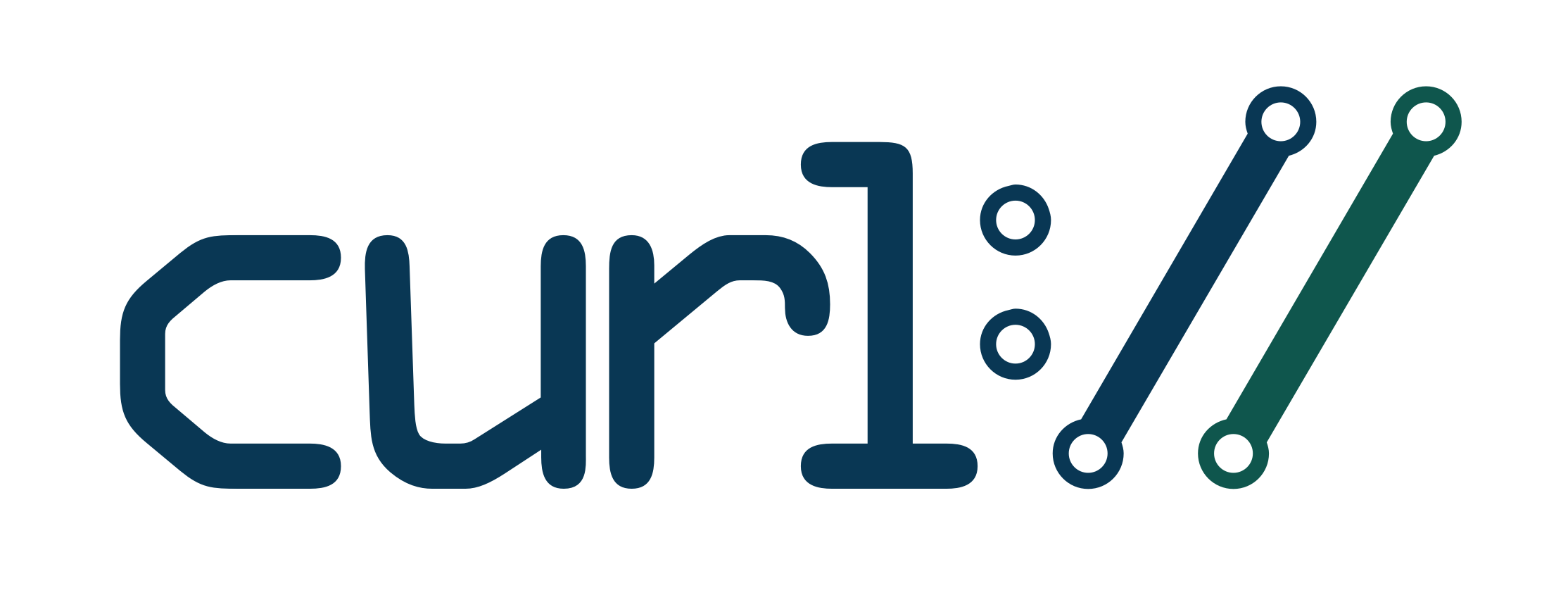
curl -G -d "order=b-11243" -d "item=shorts" -d "description=denim" -d "price=40.35" http://localhost:1555/action/orderplaced
Example Using a HTML Form
The following example is used to demonstate how an order can be placed via an external site and using your URL created in Five, the data gets entered into your database. To simulate this, a simple HTML file has been created to act as an external site. You need to enter your URL that you create in Five into the external site following their guidelines.
The example HTML file looks like below.
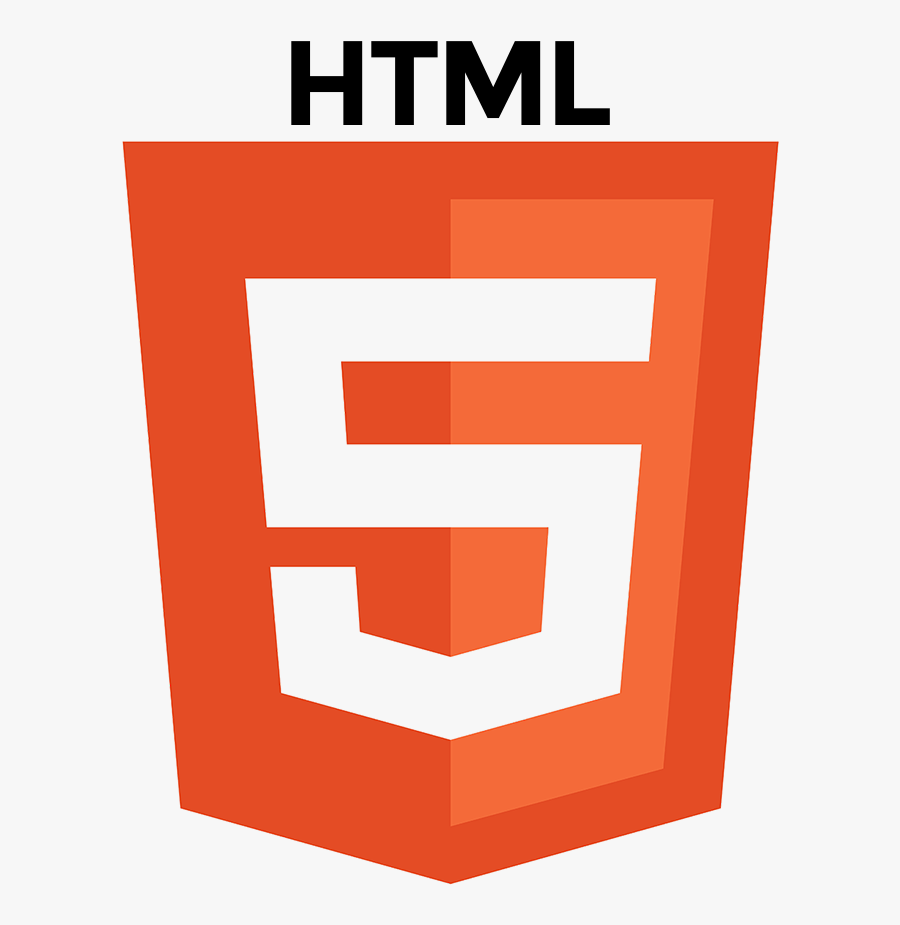
<!DOCTYPE html>
<html>
<body>
<h2>Public URL demonstration page</h2>
<form action="https://control-default-orders-example.5au.dev/action/orderplaced">
<label for="order">OrderID:</label><br>
<input type="text" id="order_id" name="order" value=""><br>
<label for="item">ItemID:</label><br>
<input type="text" id="item_id" name="item" value=""><br>
<label for="description">Description:</label><br>
<input type="text" id="description_id" name="description" value=""><br>
<label for="price">Price:</label><br>
<input type="text" id="price_id" name="price" value=""><br>
<input type="submit" value="Submit">
</form>
<p>If you click the "Submit" button, this will send a post request to the public url of your five application.</p>
</body>
</html>
It will produce a basic HTML form with input fields that will be used to simulate an external site.
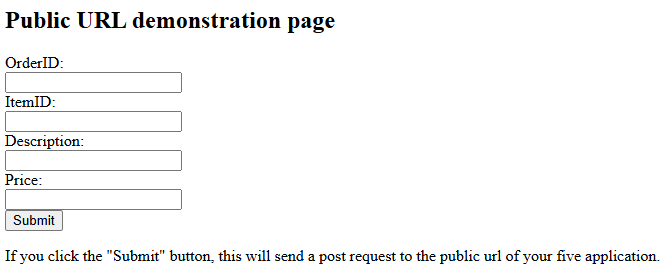
You need to have your table created in Five to store the data, this table needs the Create permission for the Public role as URLs are executed under the Public user. For this example, the table has the same fields as the HTML form and a primary key field to store a GUID generated by Five. A form or data view can be created to display these results in your application. It is decided by you if the results in a form can be updated, deleted, or in a read-only format.
Add Your Function
The example function is called OrderPlaced and takes the details to place an order that have been provided as URL encoded parameters.
The
The input name is the name of the input field in the HTML form above.
The
The
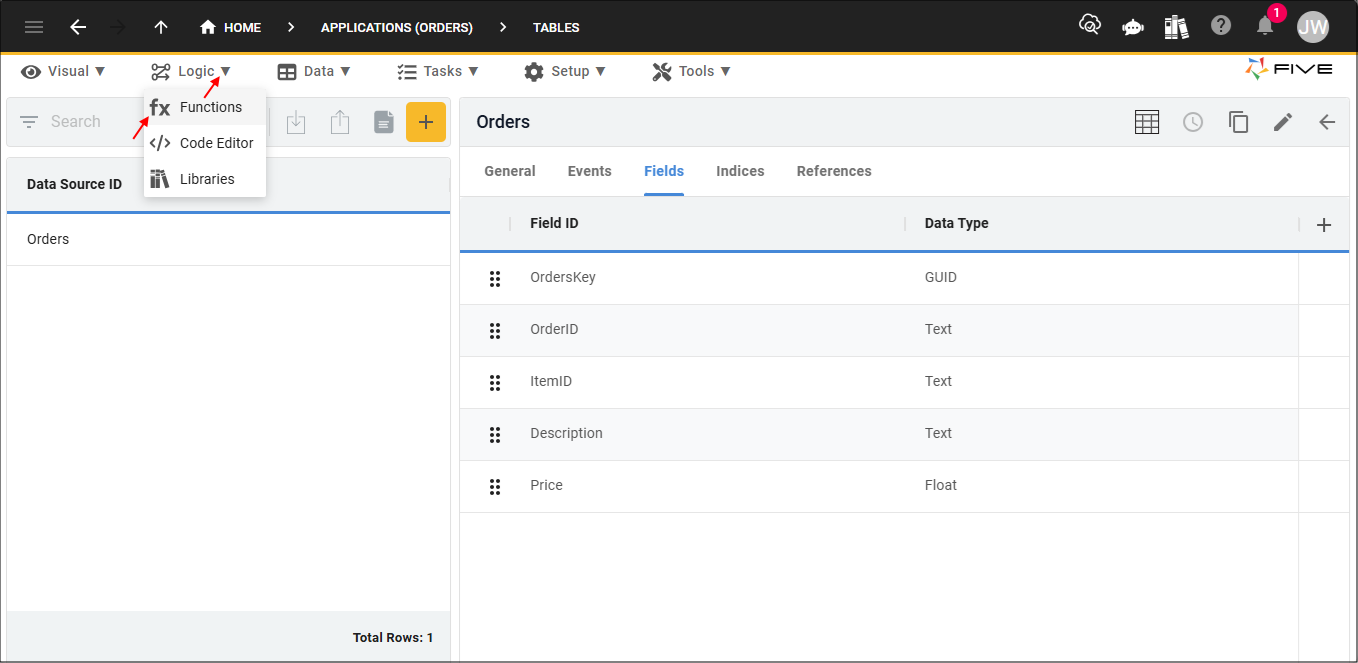
2. Click the Add Item button and type an ID in the Function ID field.
3. Click in the Code field.
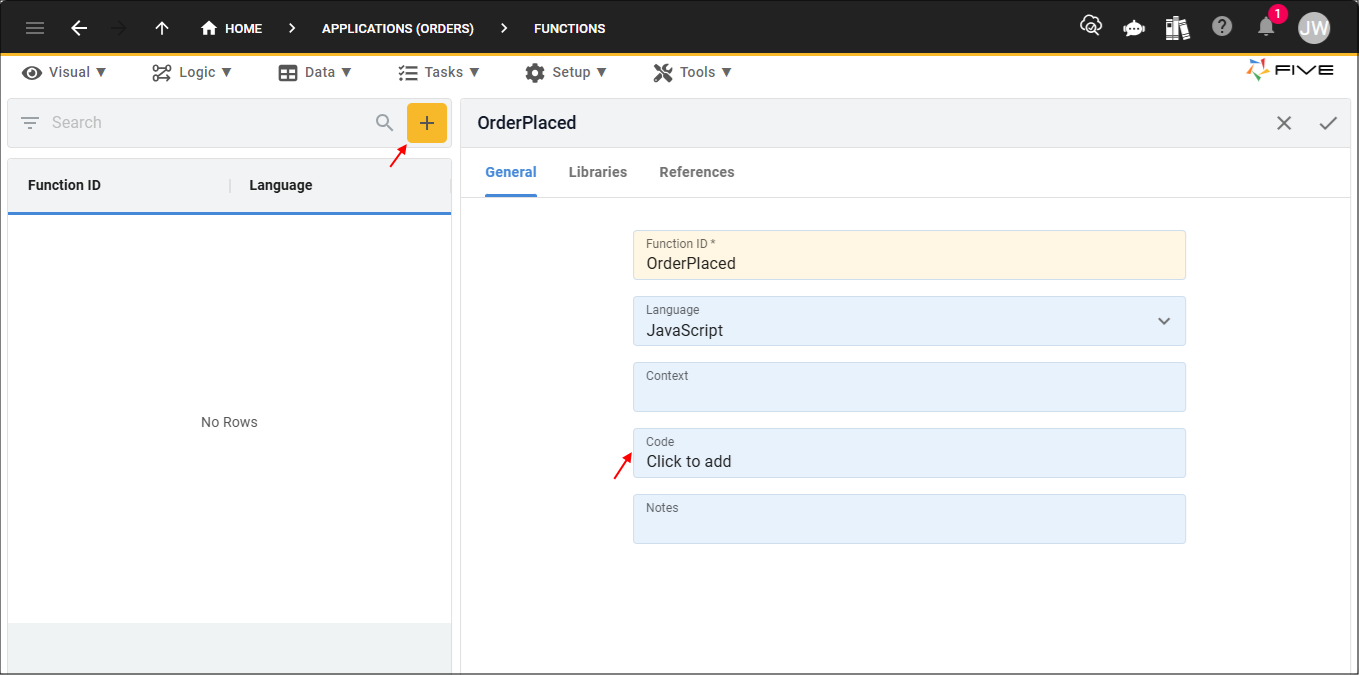
4. Add your function following the example code below.
function OrderPlaced(five, context, result) {
const order = five.parameters.order;
const description = five.parameters.description;
const price = five.parameters.price;
const item = five.parameters.item;
const sql = 'INSERT INTO Orders (OrdersKey, OrderID, ItemID, Description, Price) VALUES (?,?,?,?,?)'
let queryResults = five.executeQuery(sql, 0, five.uuid(), order, item, description, price);
if(queryResults.isOk() === false){
return five.createError(queryResults, "Failed to insert record");
}
return five.success();
}
5. Click the Save button in the code editor.
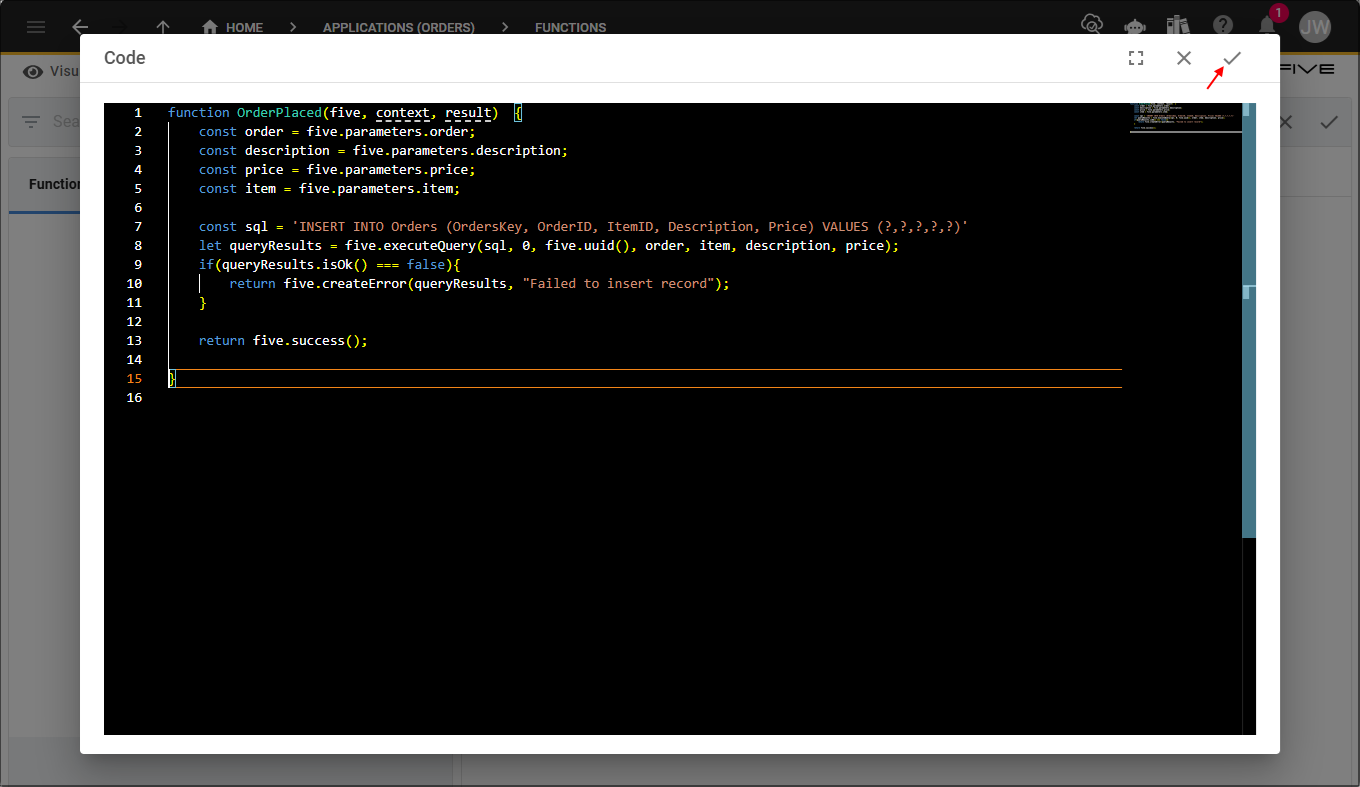
6. Click the Save button in the form app bar.
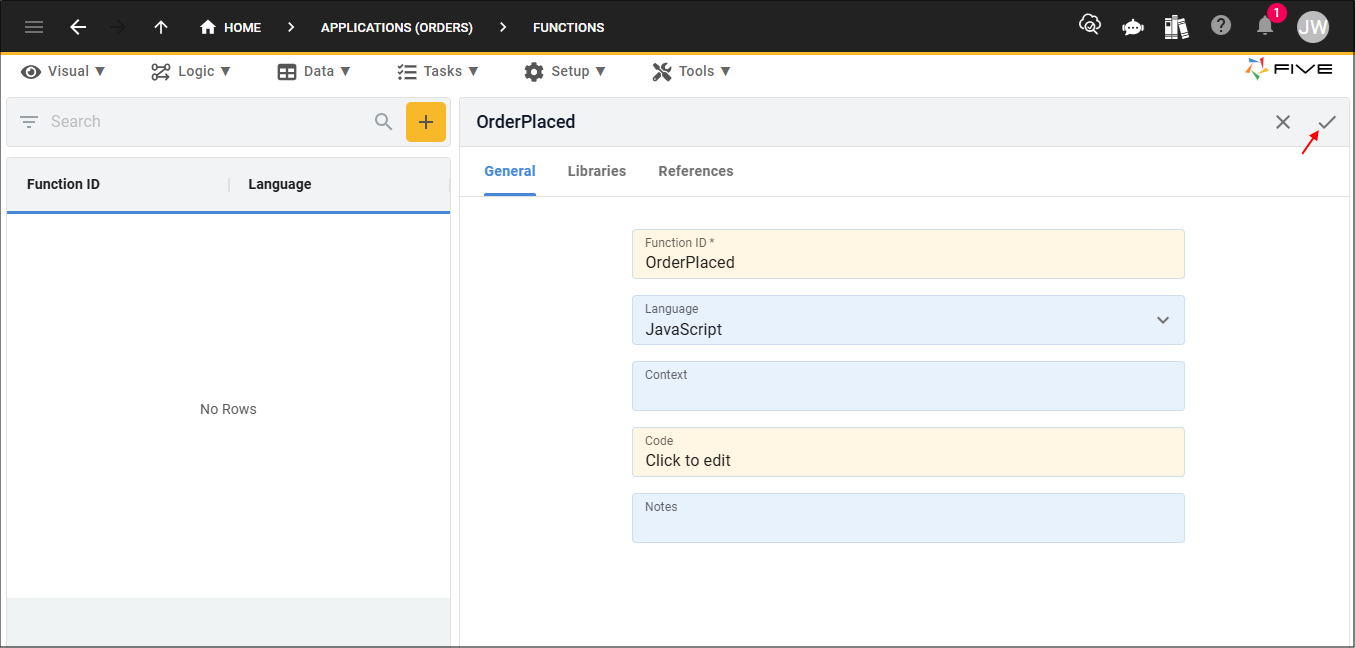
Add Your Process
The process enables the
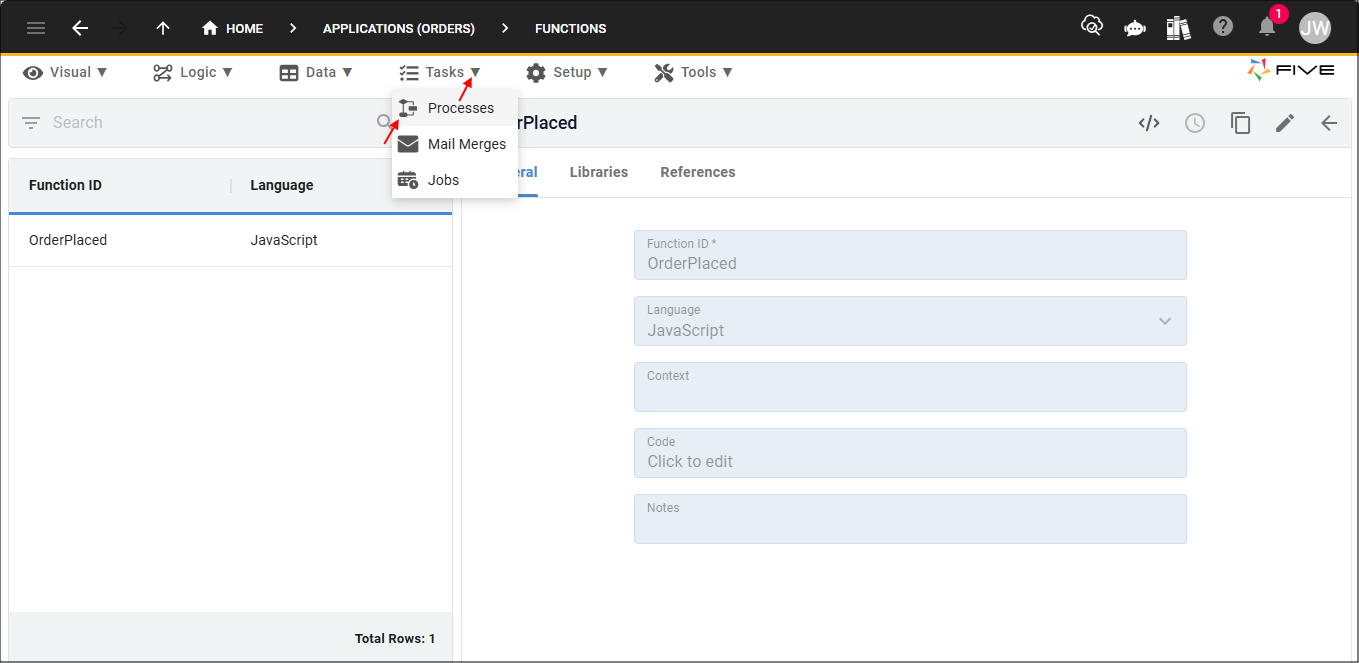
2. Click the Add Item button and type a title in the Title field.
3. Click the Background button.
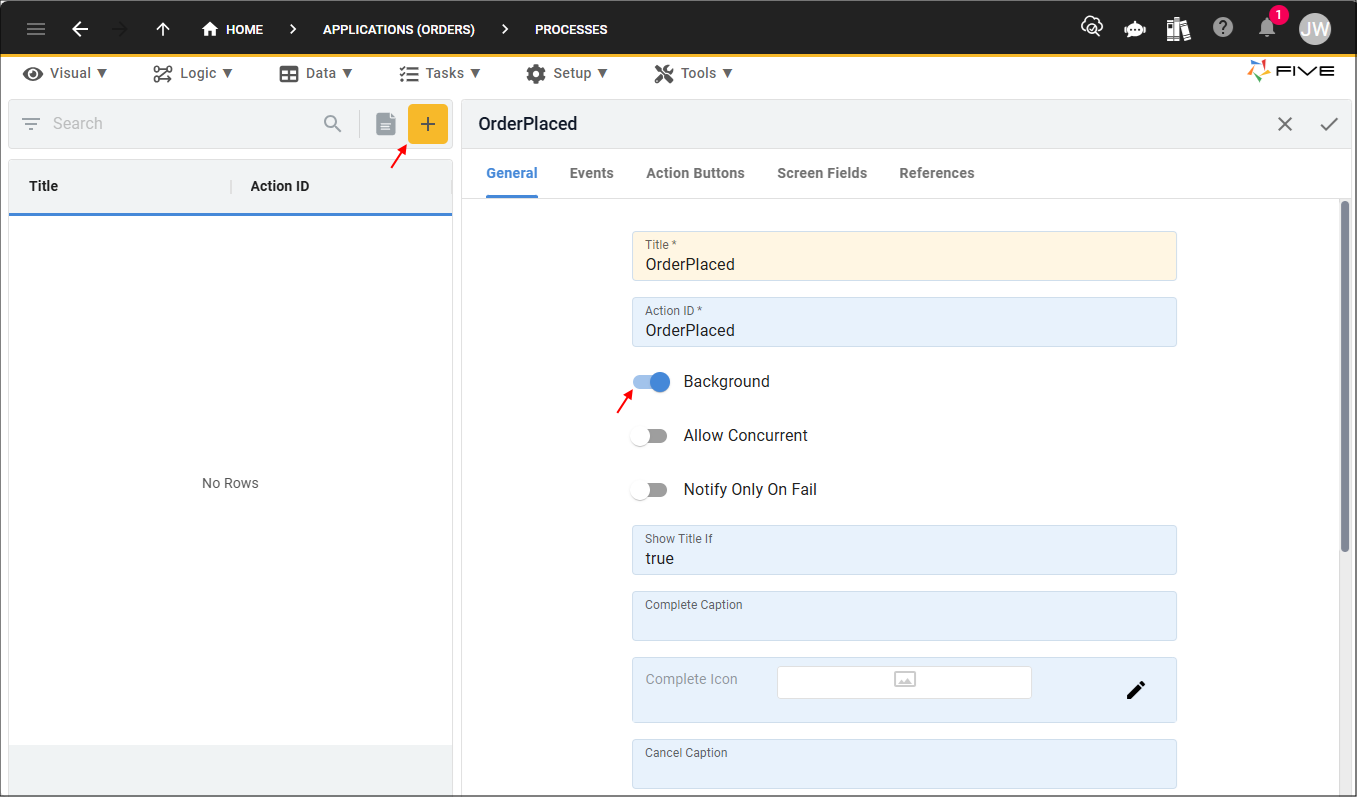
4. Click the Events tab.
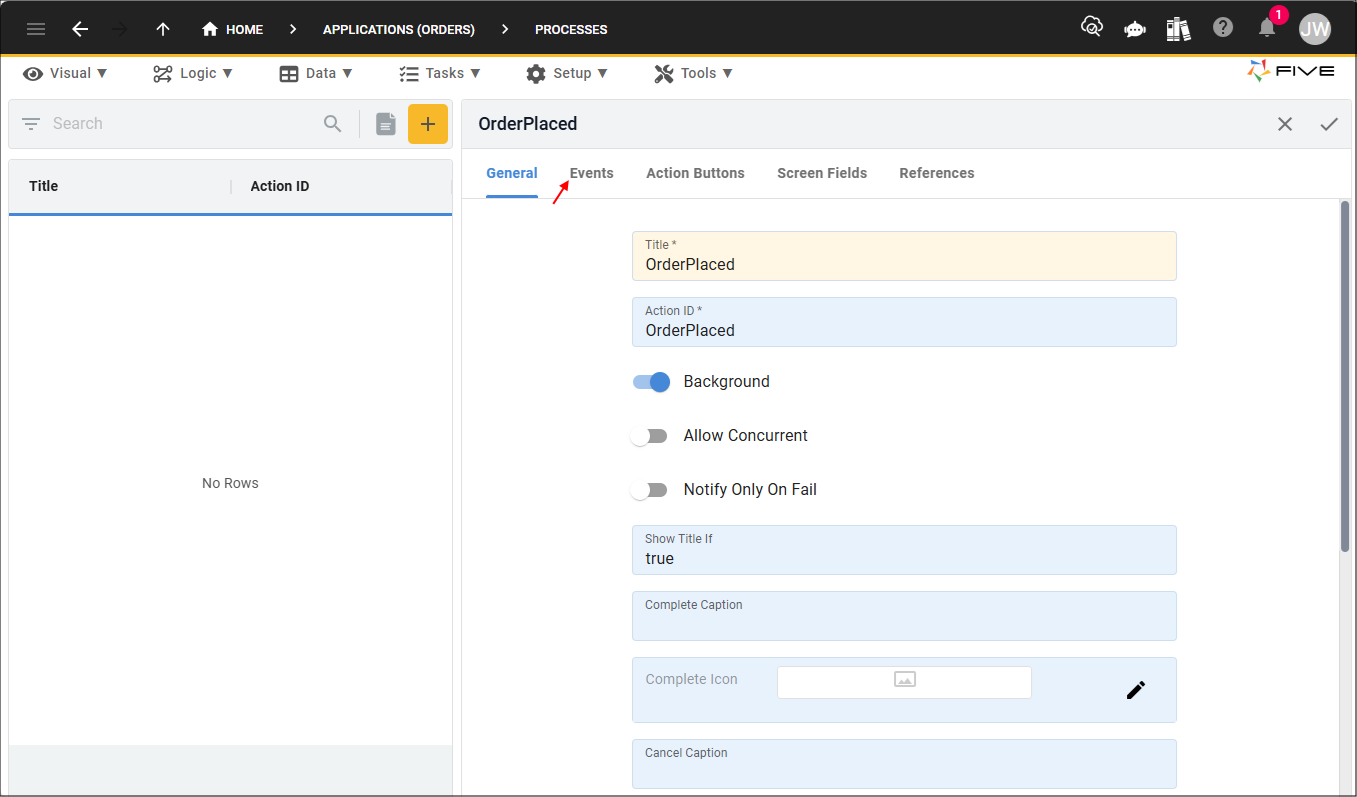
5. Click the lookup icon in the Do Run field and select your function.
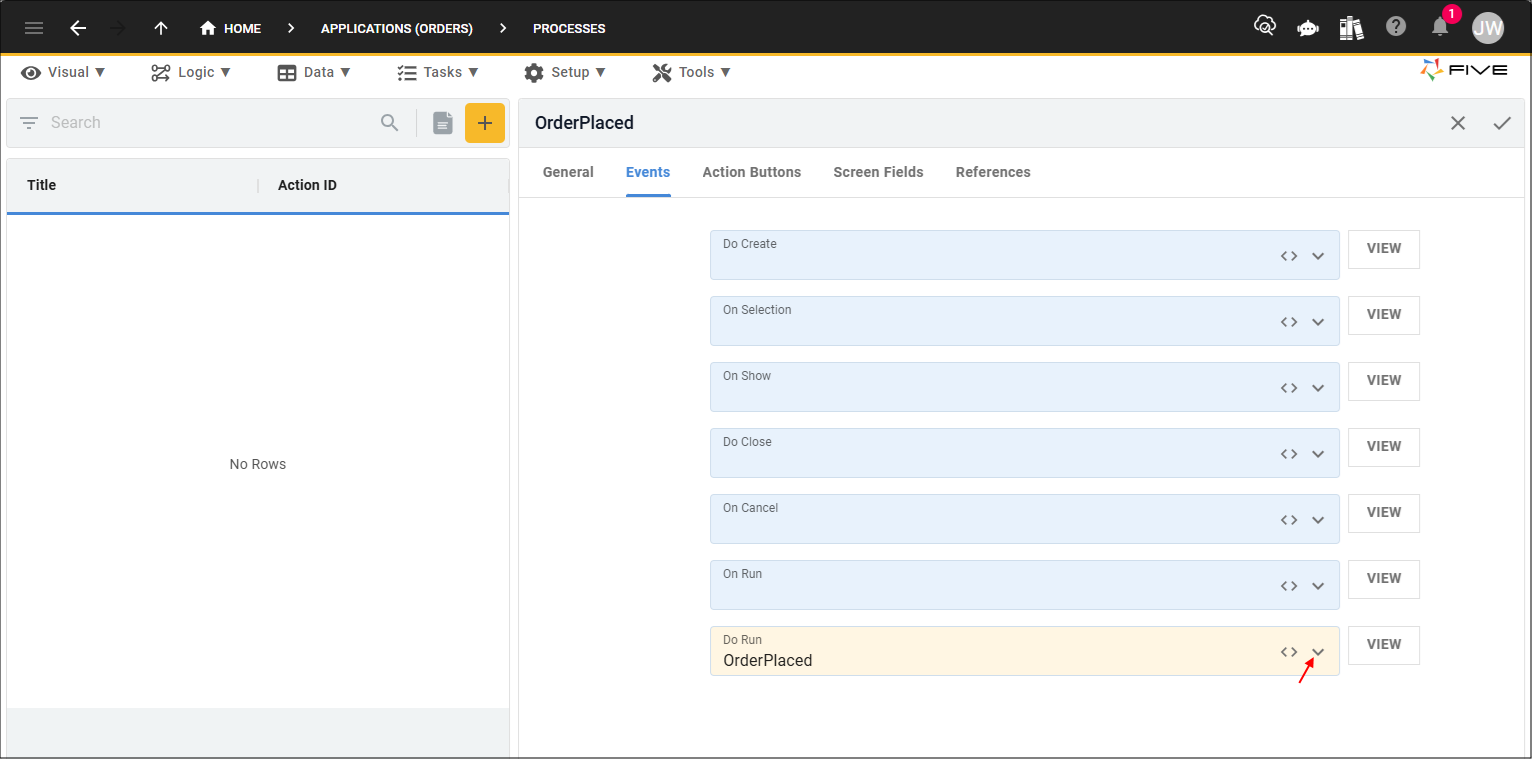
6. Click the Save button in the form app bar.
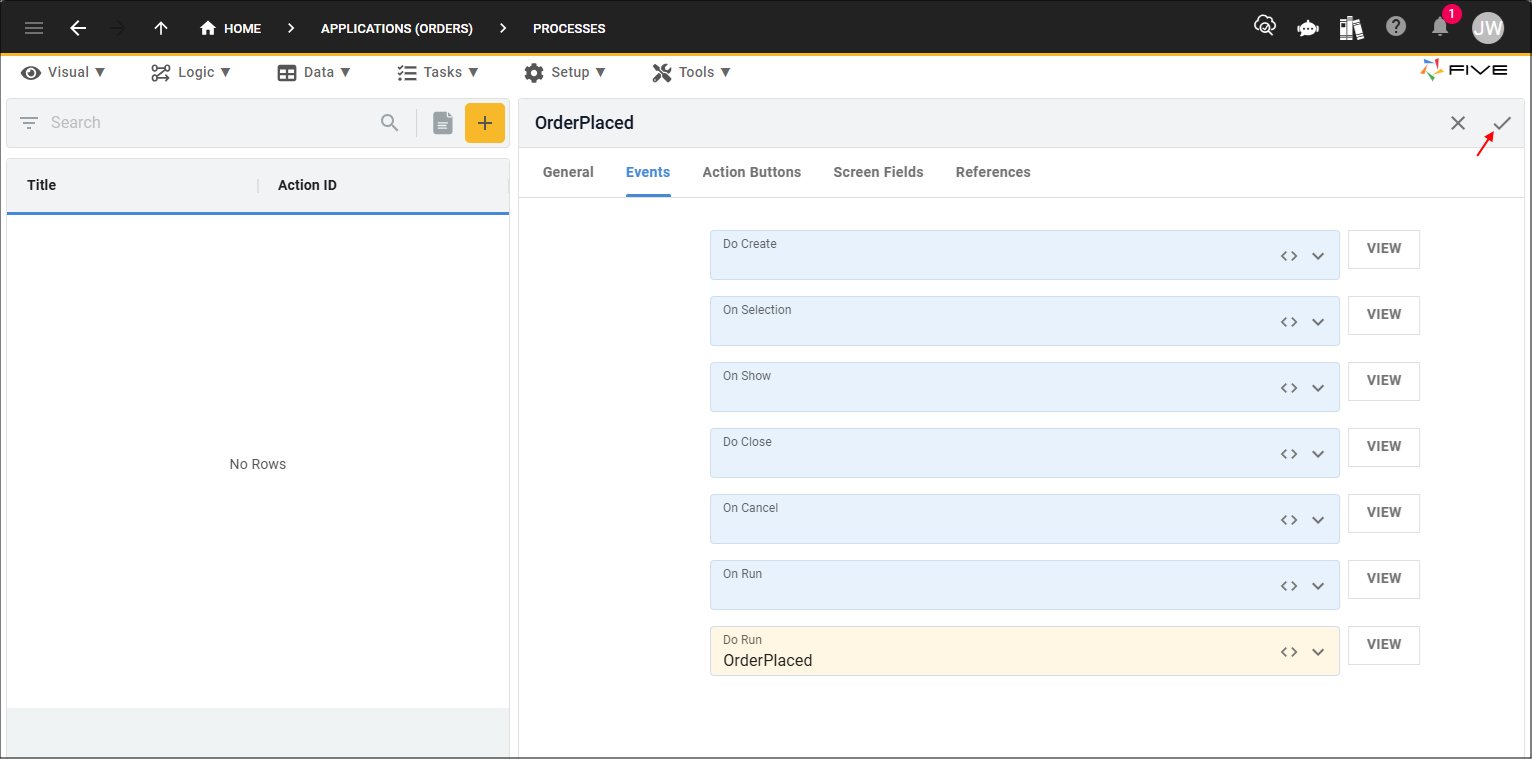
Add Your URL
Your URL will reference your process so that when the data has been submitted from your external site, your URL created in Five will be used to receive the data and insert it into your database. Using the example HTML file ensure the form host name is your Five's host name.
Examplehttps://control-default-orders-example.5au.dev/action/orderplaced
1. Select Setup in the menu followed by URLs in the sub-menu.
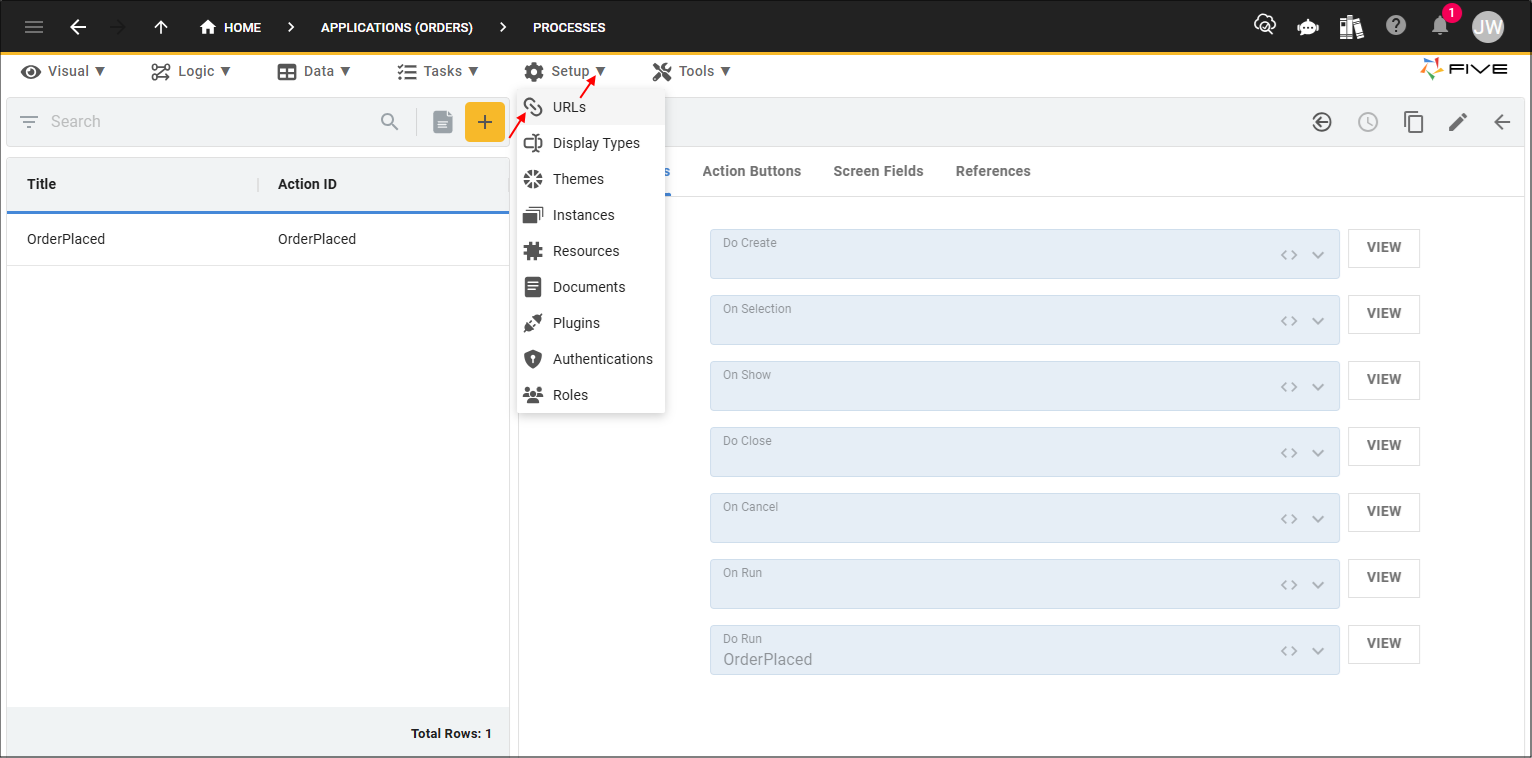
2. Click the Add Item button and type a name in the URL field.
3. Click the lookup icon in the Action field and select your process.
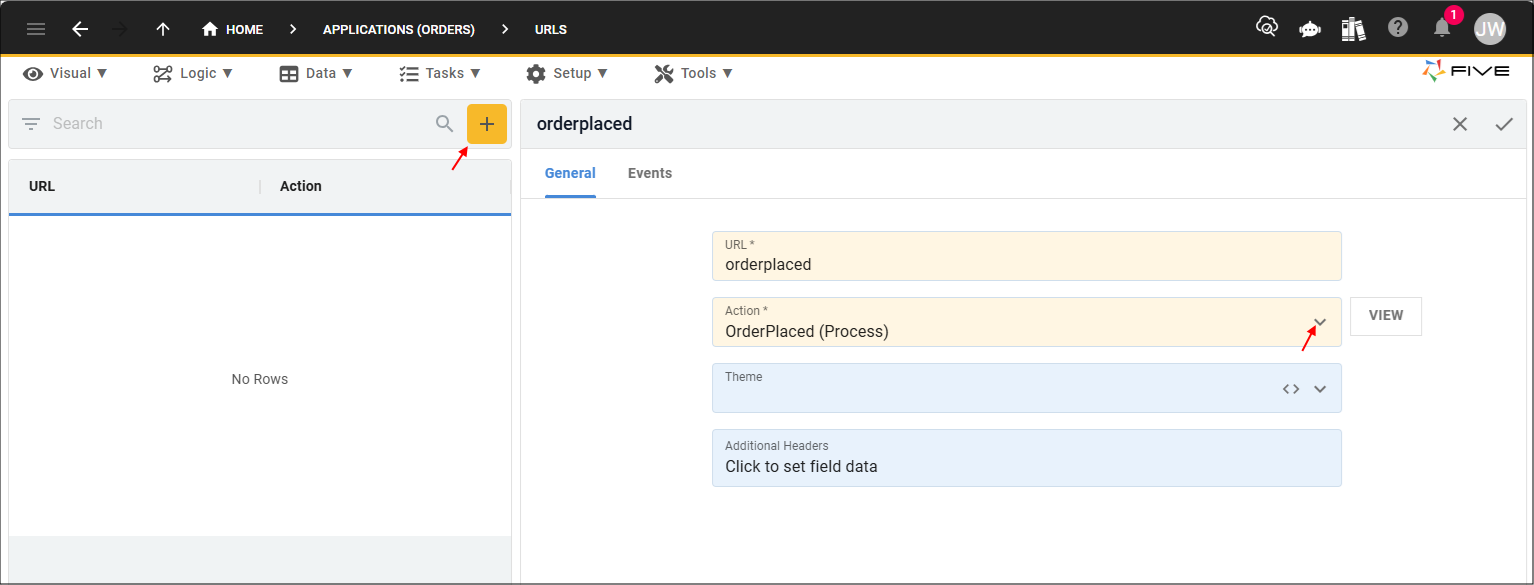
4. Click the Save button in the form app bar.
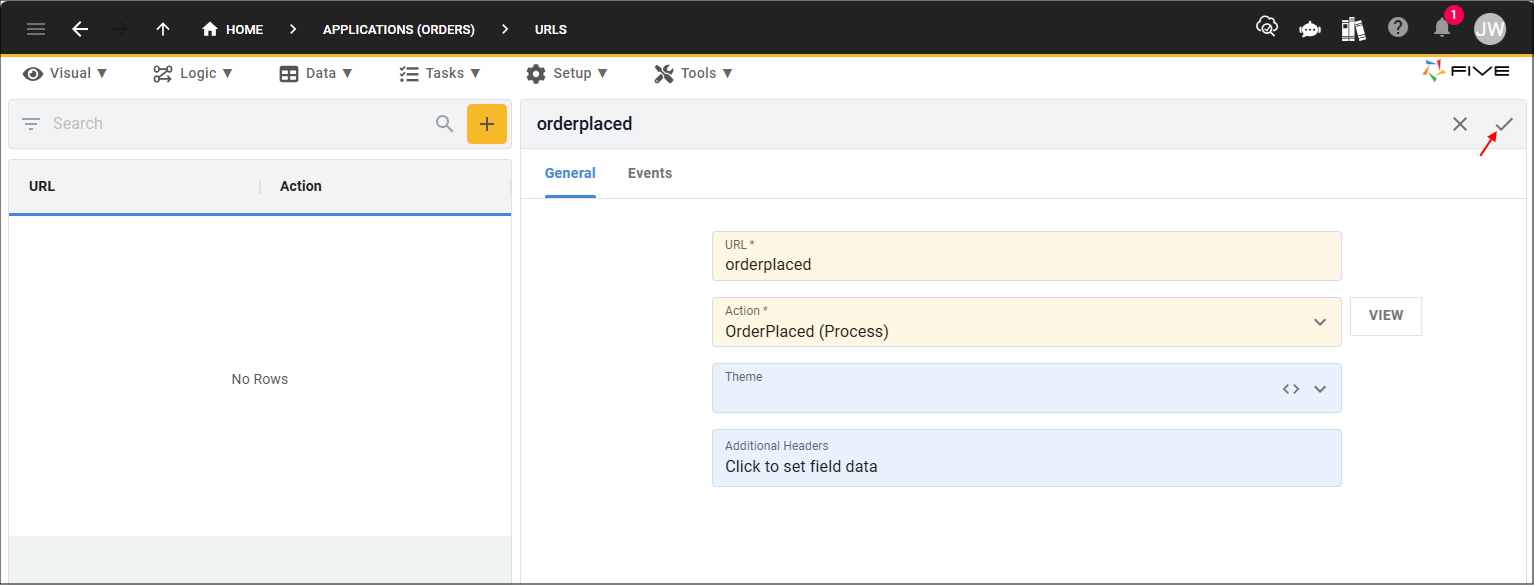
Display the Results
You can run your URL and have the process run completely behind the scenes and have the data populated into your table/s. If you wish to display the results you can create a data view and this will display the records which cannot be edited.
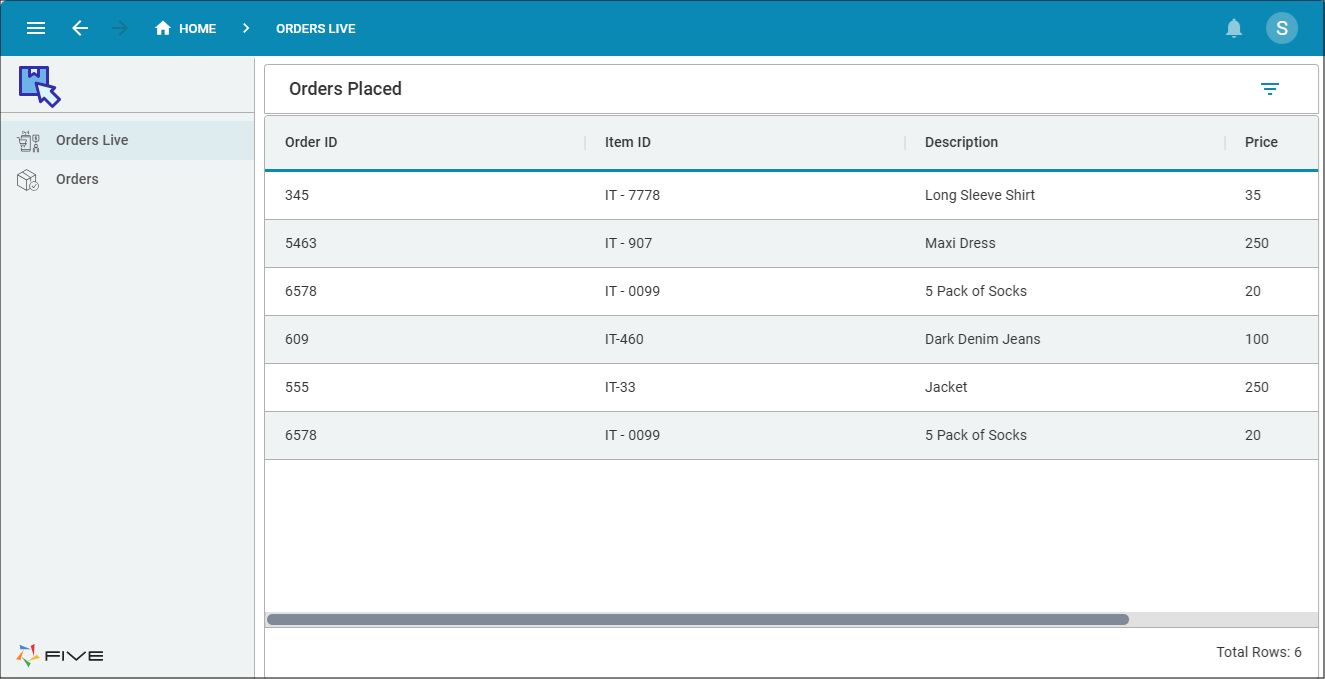
Alternatively, you can create a form which can be set to read-only if required.
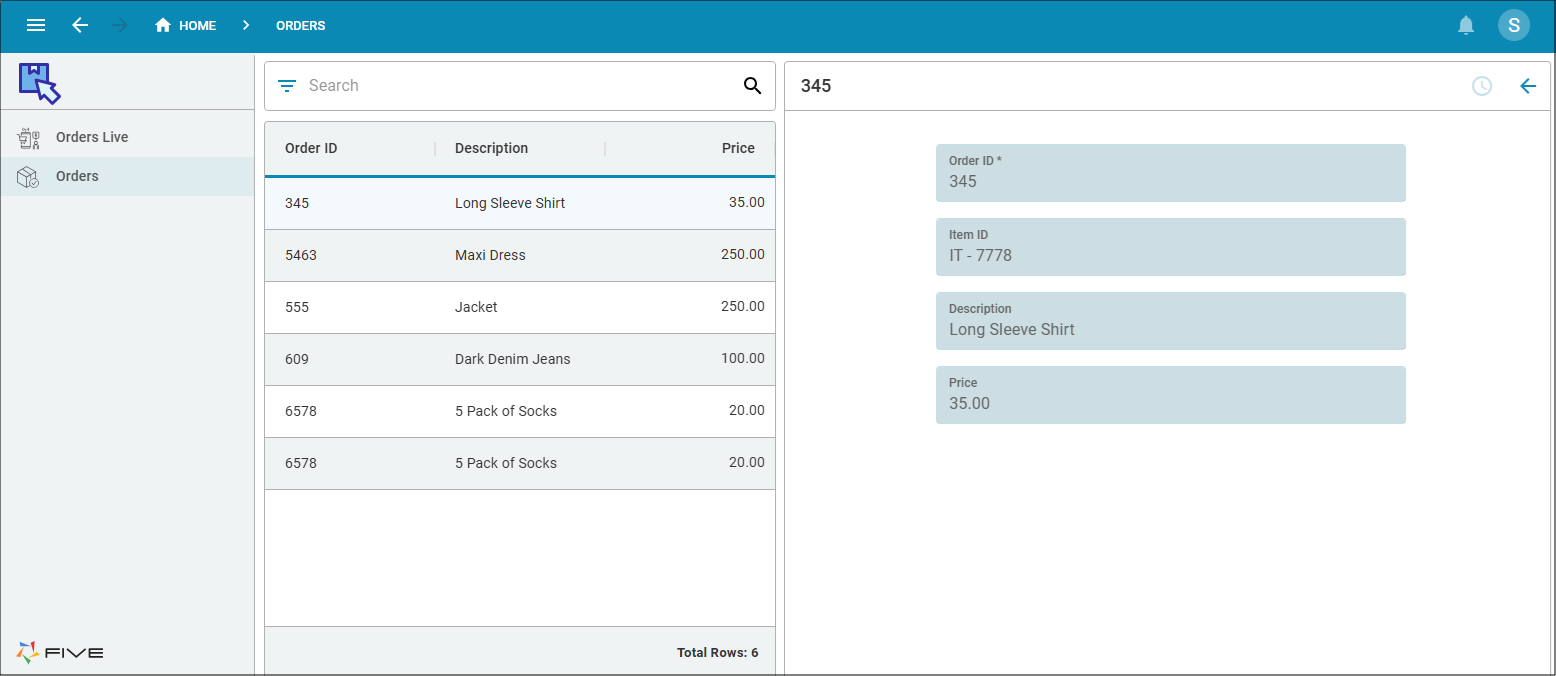