9 - Call a Server-Side Function From the Client
Last updated 4/04/2023
This documentation is to demonstrate how you can add a function that executes in the browser and calls a function in the backend to interact with the database.Problem
We have another problem we need to rectify in the Portfolio application and this can be performed by having the client call the backend to verify the data.
Currently in the Portfolio application, when a purchase is made there is no warning that you may be buying under the low price or over the high price for the day.
In Five, we will create two functions, the CheckPrice function will execute in the browser and call the CheckPriceServer function from the backend to get the results from the database so we can display a message alerting that the buy price is lower or higher than the daily price.
Currently in the Portfolio application, when a purchase is made there is no warning that you may be buying under the low price or over the high price for the day.
In Five, we will create two functions, the CheckPrice function will execute in the browser and call the CheckPriceServer function from the backend to get the results from the database so we can display a message alerting that the buy price is lower or higher than the daily price.
Navigate to the Code Editor
1. Select Logic in the menu.2. Select Code Editor in the sub-menu.
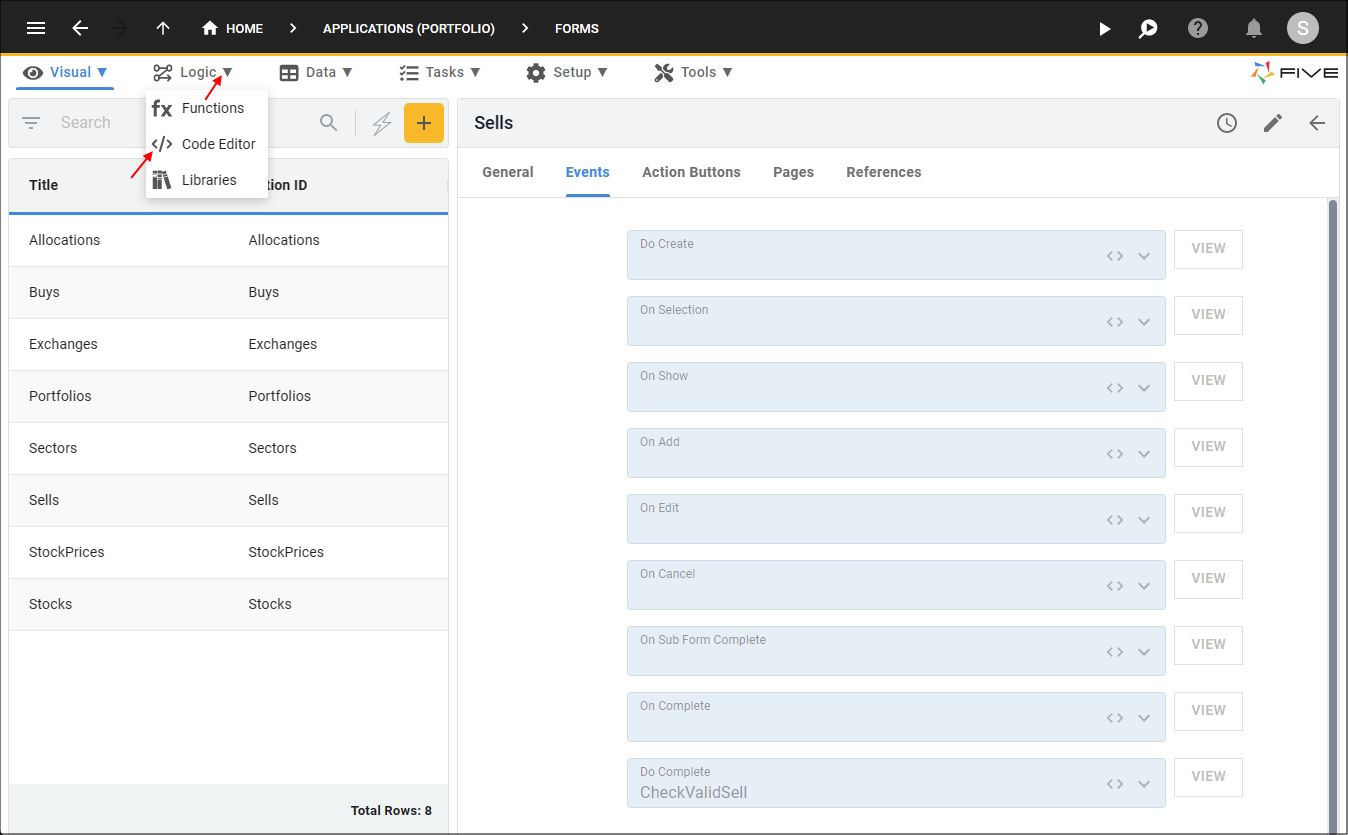
Figure 1 - Code Editor menu item
Add the CheckPriceServer Function
1. Click the Add New Code button.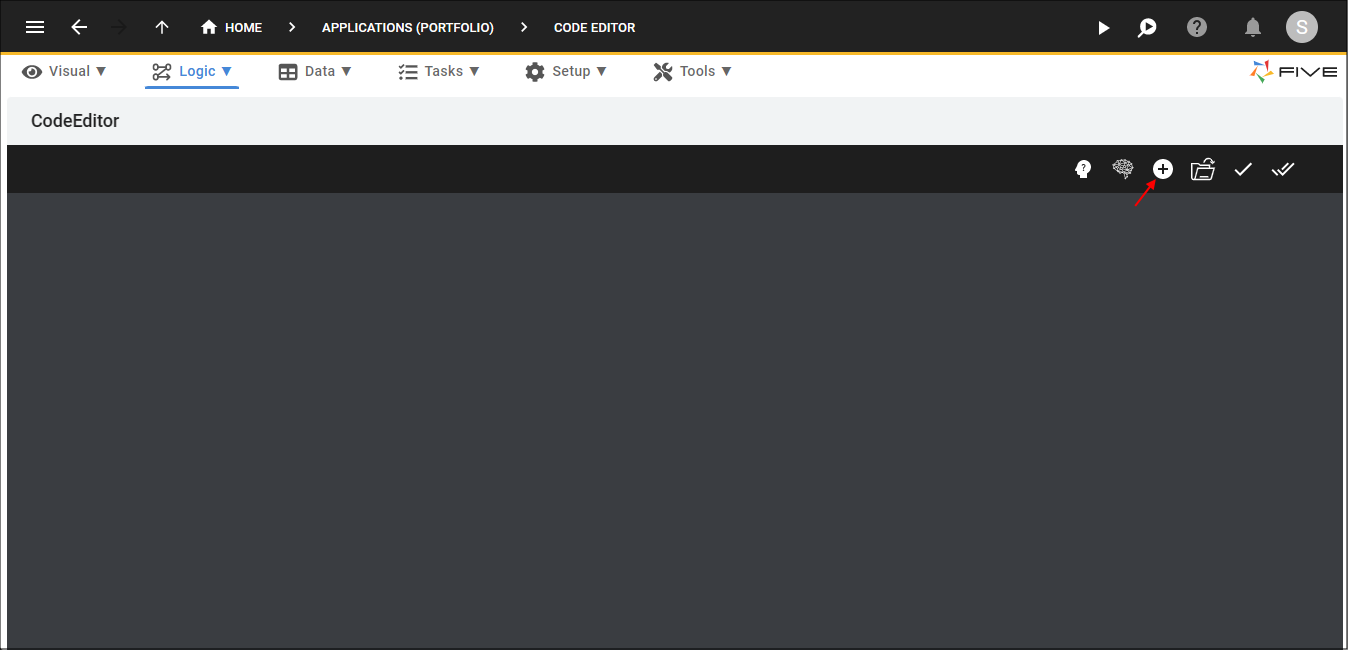
Figure 2 - Add New Code button
2. Type CheckPriceServer in the Function ID field.
3. Click the lookup icon in the Language field and select TypeScript.
4. Click the OKAY button.
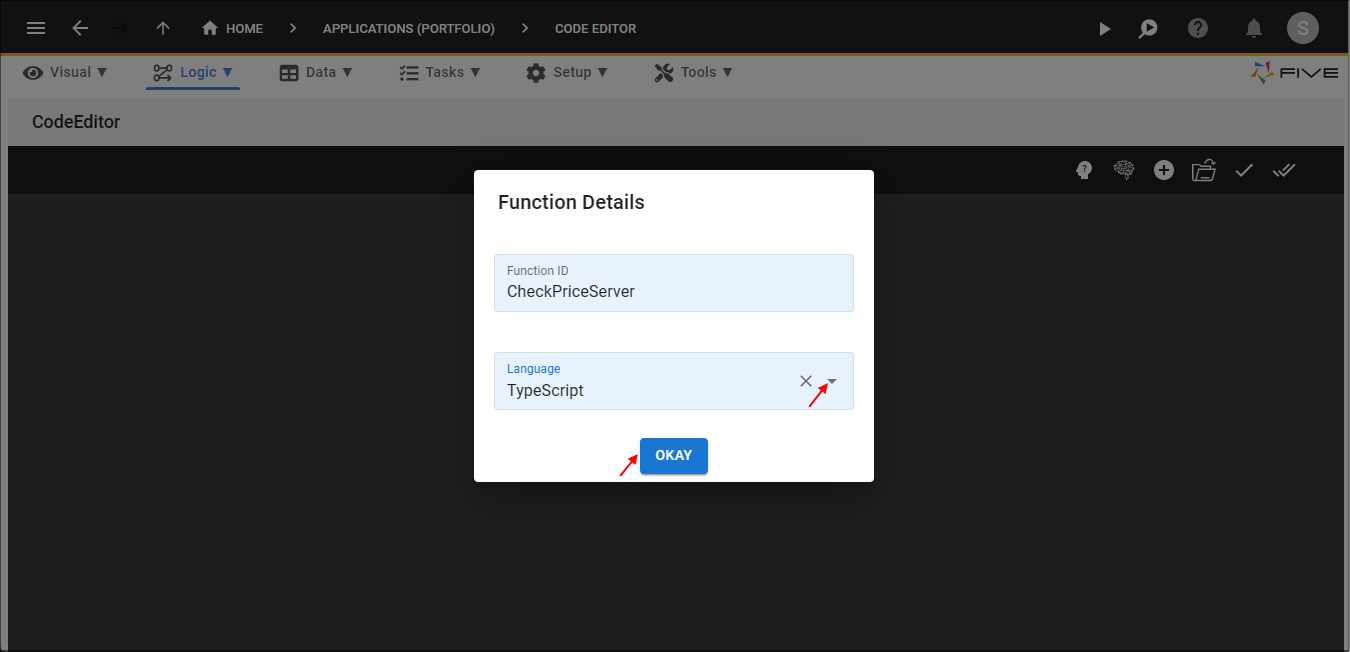
Figure 3 - Add the CheckPriceServer function
5. Click the Copy button on the below code block.
CheckPriceServer
function CheckPriceServer(five: Five, context: any, result: FiveError) : FiveError {
const sqlStatement: string = "SELECT High, Low FROM StockPrice WHERE StockKey = ? AND PriceDate = ?";
const queryresults: QueryResult = five.executeQuery(sqlStatement, 0, context.StockKey, context.TransactionDate);
if (queryresults.values === null) {
return five.success(result);
}
const highPrice: number = queryresults.values[0].High;
const lowPrice: number = queryresults.values[0].Low;
const price: number = context.Price;
let message = '';
if ((highPrice === null) || (lowPrice === null)) {
return five.success(result);
} else {
if (Number(price) < Number(lowPrice)) {
message = 'Price is lower than price low for the day';
} else if (Number(price) > Number(highPrice)){
message = 'Price is higher than price high for the day';
} else {
return five.success(result);
}
}
return five.createError(result, message)
}
6. Paste the code block over the template in the Code Editor.
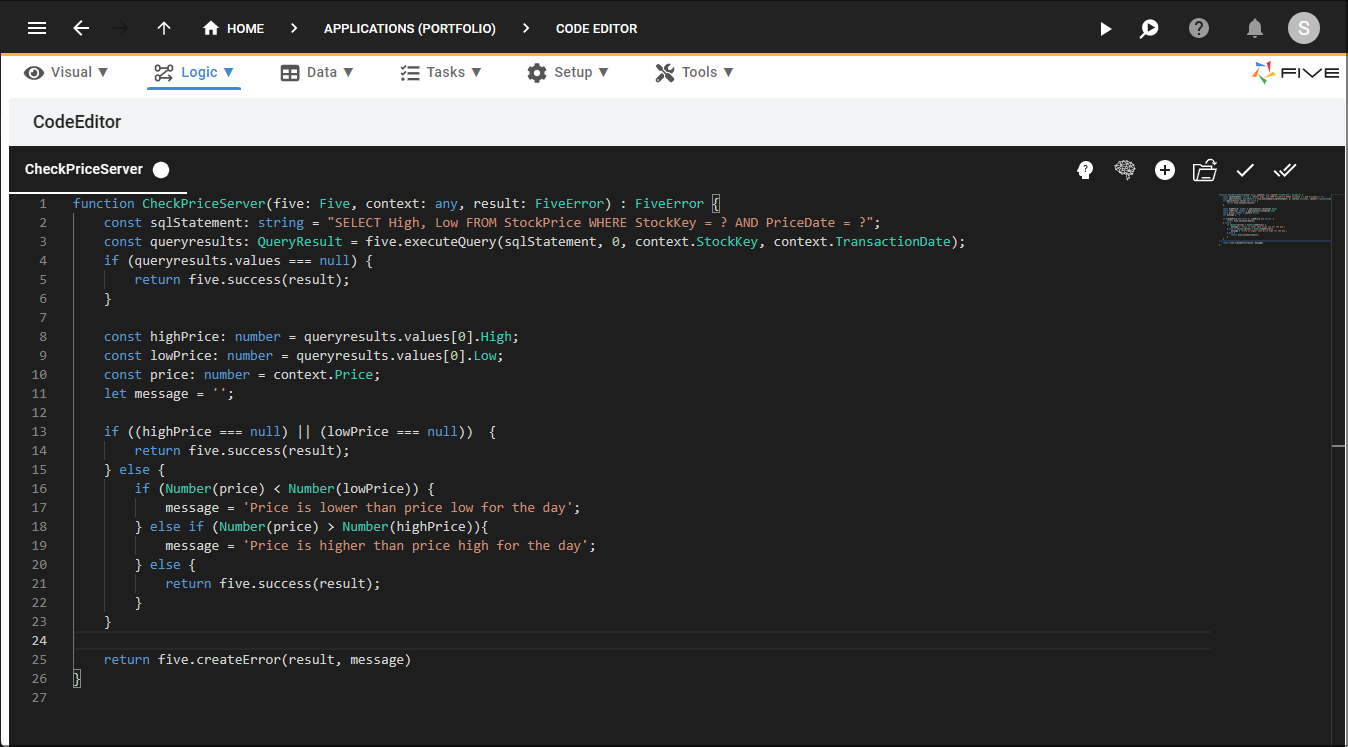
Figure 4 - CheckPriceServer code
Add the CheckPrice Function
1. Click the Add New Code button.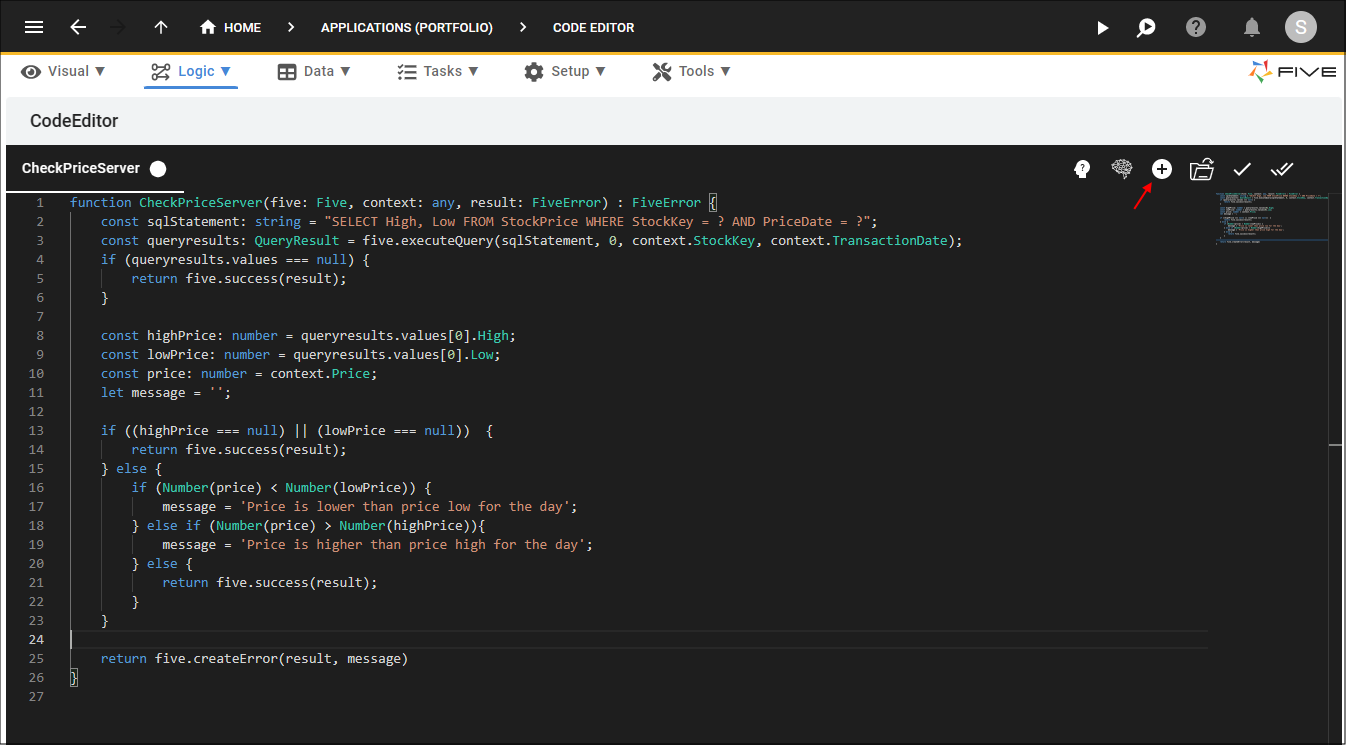
Figure 5 - Add New Code button
2. Type CheckPrice in the Function ID field.
3. Click the lookup icon in the Language field and select TypeScript.
4. Click the OKAY button.
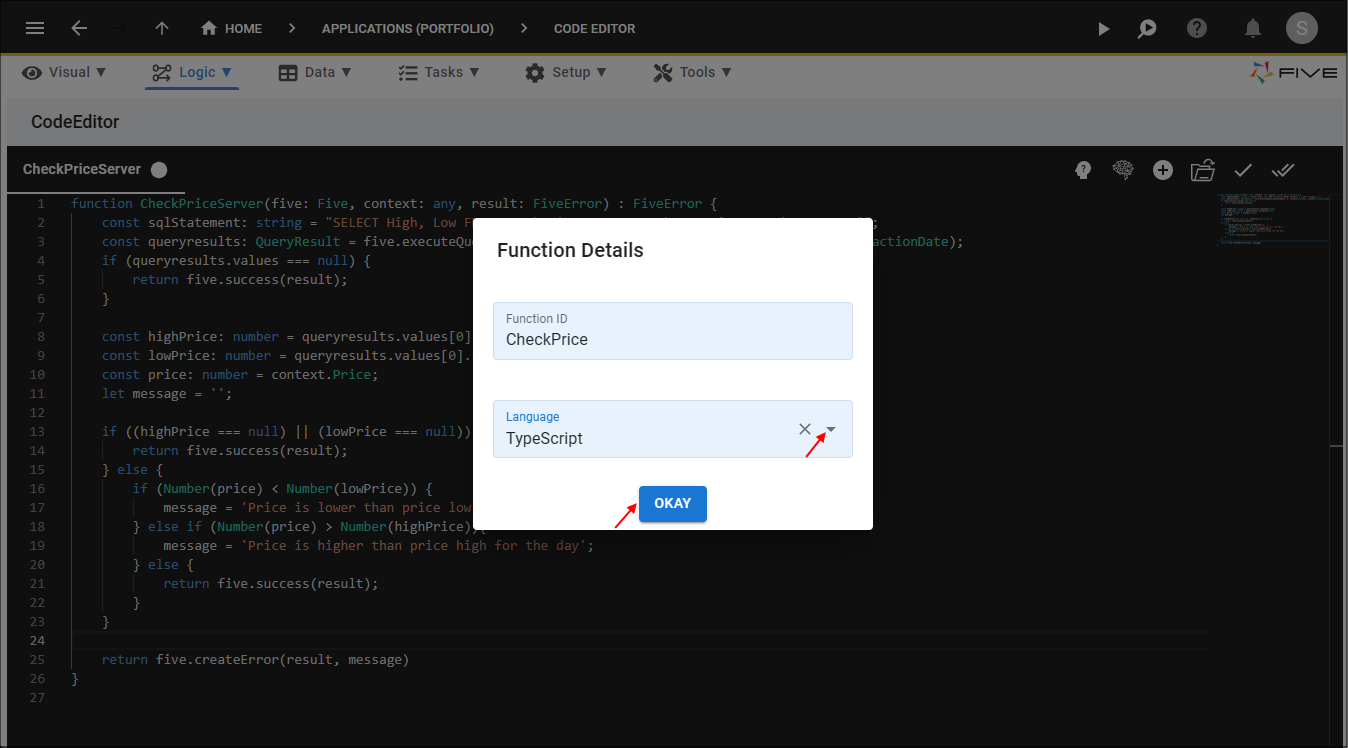
Figure 6 - Add the CheckPrice function
5. Click the Copy button on the below code block.
CheckPrice
function CheckPrice(five: Five, context: any, result: FiveError) : FiveError {
const functionKey: string = '';
const applicationKey: string = '';
const selectedFile: any = null;
const functionName: string = 'CheckPriceServer';
const variables: any = {}
variables['StockKey'] = five.field.StockKey;
variables['TransactionDate'] = five.field.TransactionDate;
variables['Price'] = five.field.Price;
const _five: Five = five;
five.executeFunction(functionName, variables, selectedFile, functionKey, applicationKey, function (result) {
if (result.serverResponse.errorCode === 'ErrErrorOk') {
return;
}
const functionMessage = result.serverResponse.results;
if (functionMessage !== '') {
_five.showMessage(functionMessage);
}
});
return five.success(result);
}
6. Paste the code block over the template in the Code Editor.
7. Click the Save All Tabs button
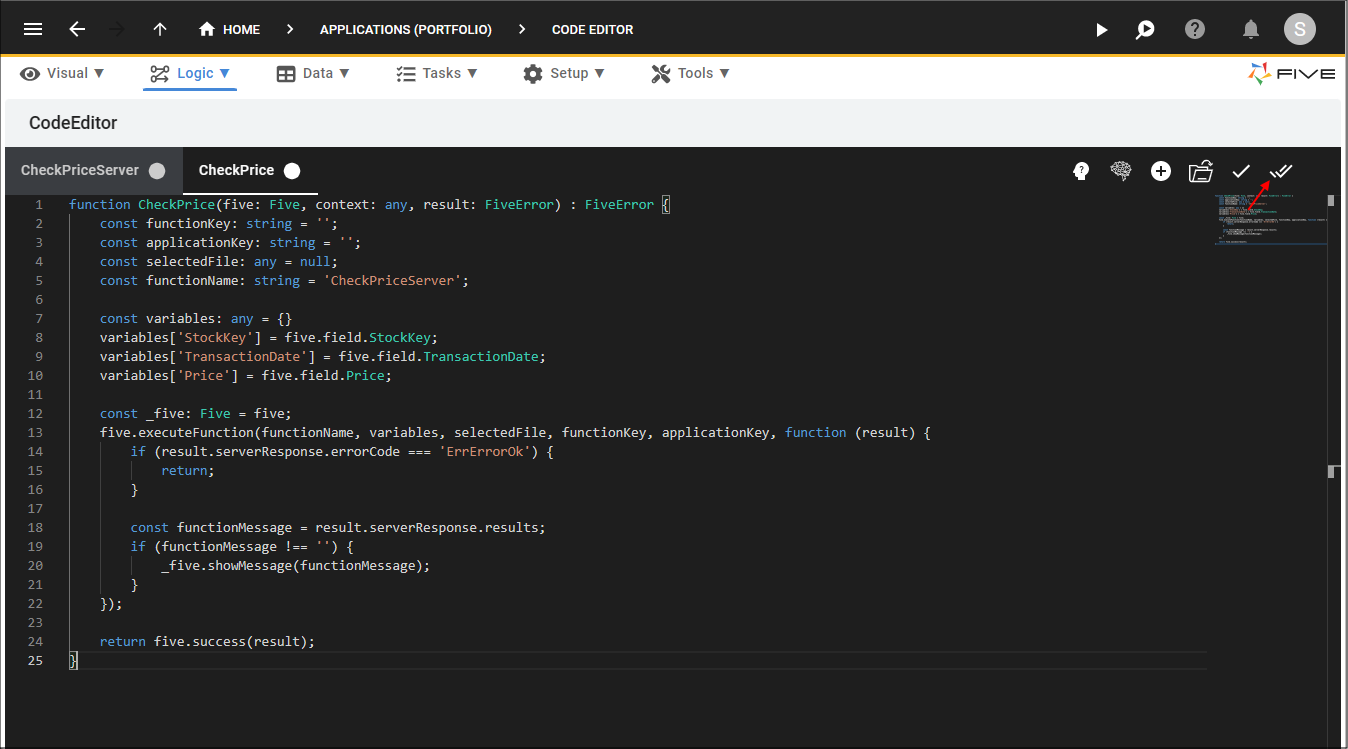
Figure 7 - Save All Tabs button
Attach the CheckPrice Function
1. Select Visual in the menu.2. Select Forms in the sub-menu.
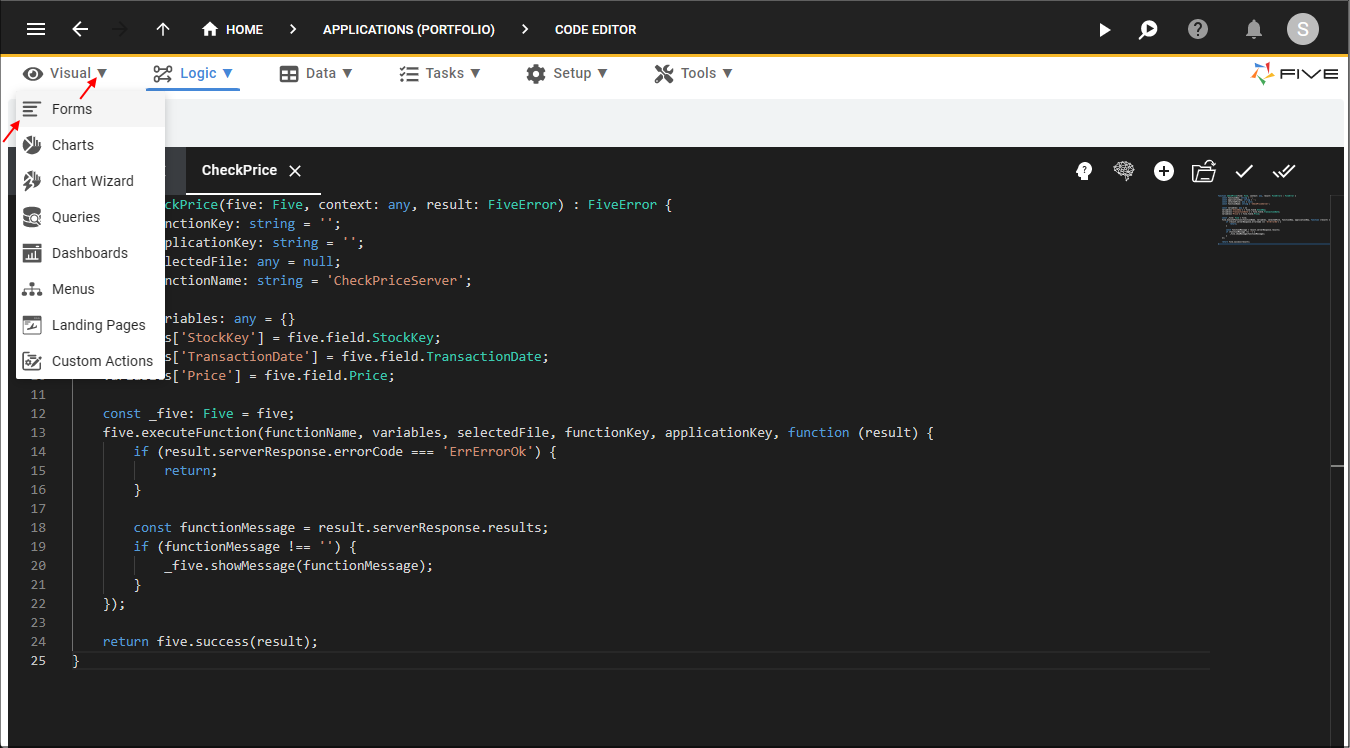
Figure 8 - Forms menu item
3. Select the Buys record in the list.
4. Click the Pages tab.
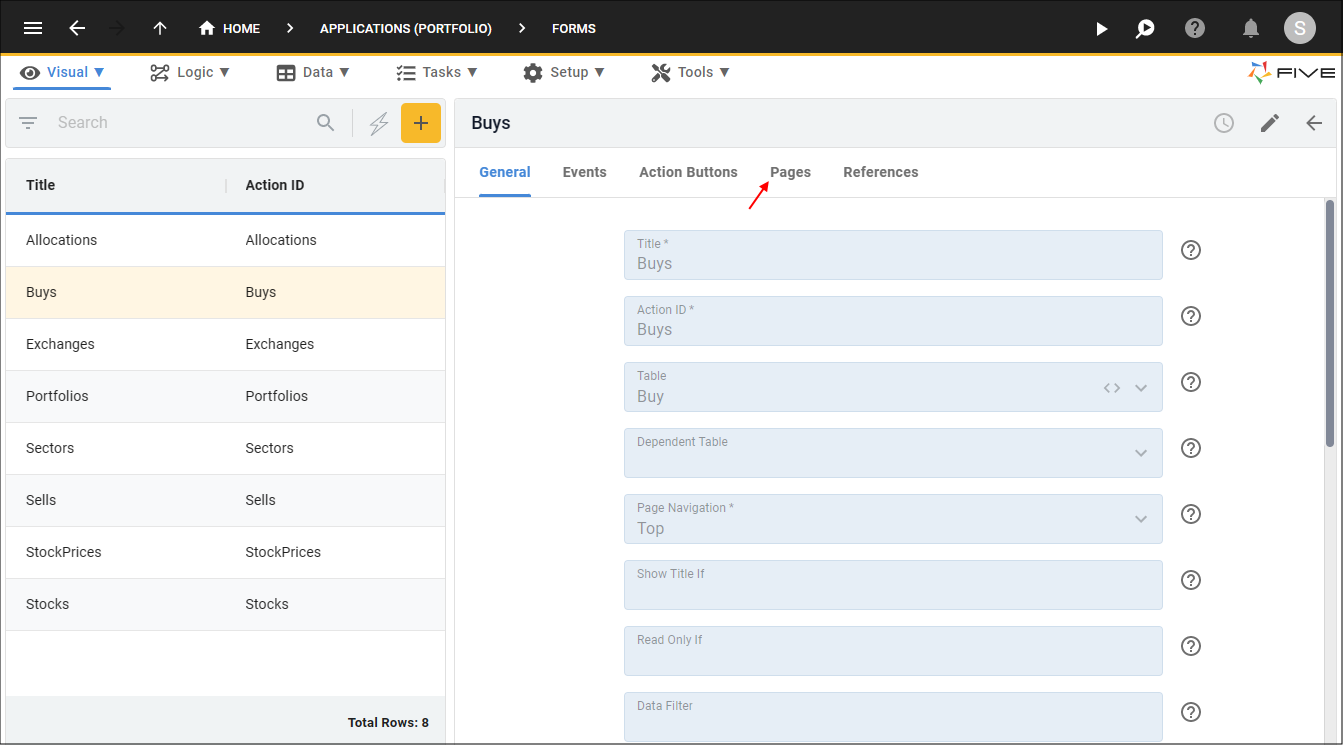
Figure 9 - Pages tab
5. Select the General record.
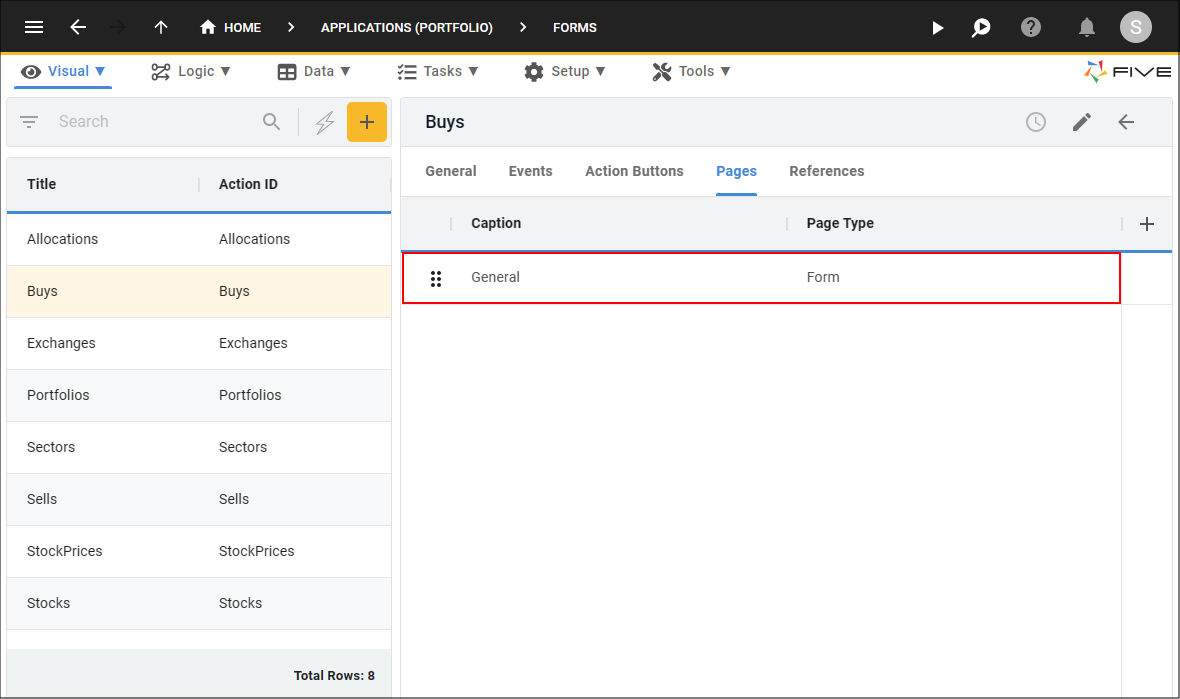
Figure 10 - General record
6. Click the Fields tab.
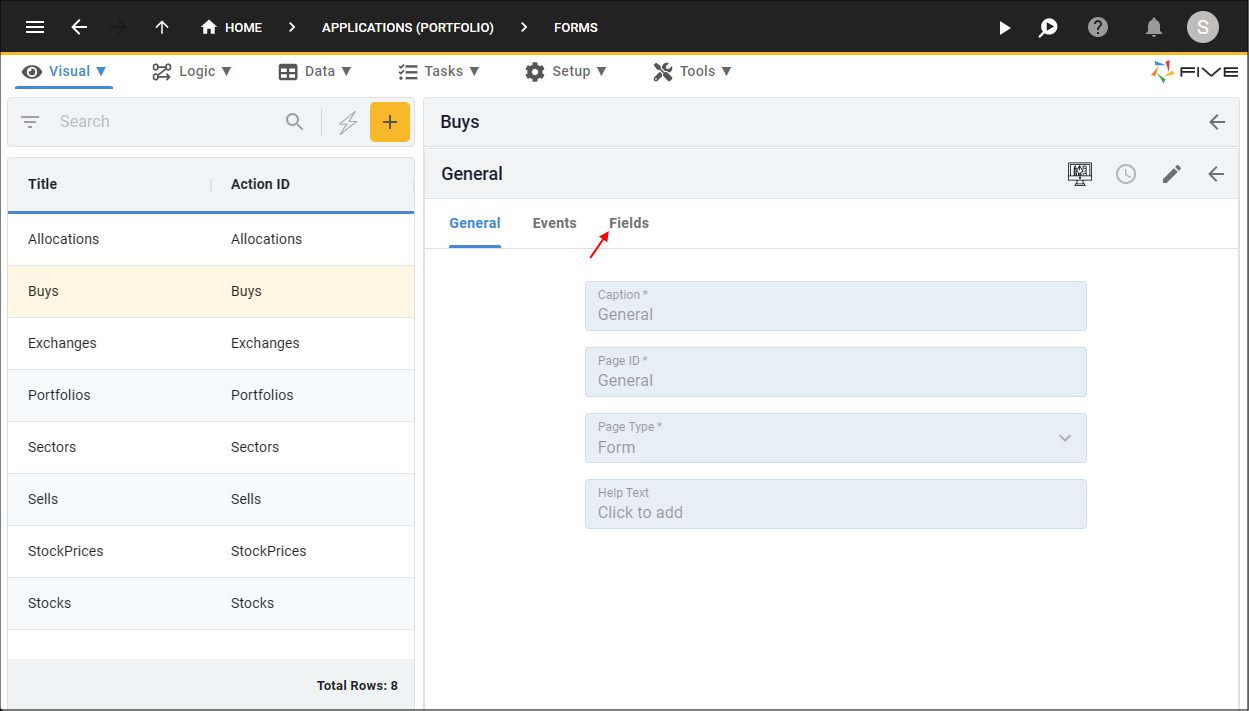
Figure 11 - Fields tab
7. Select the Price record.
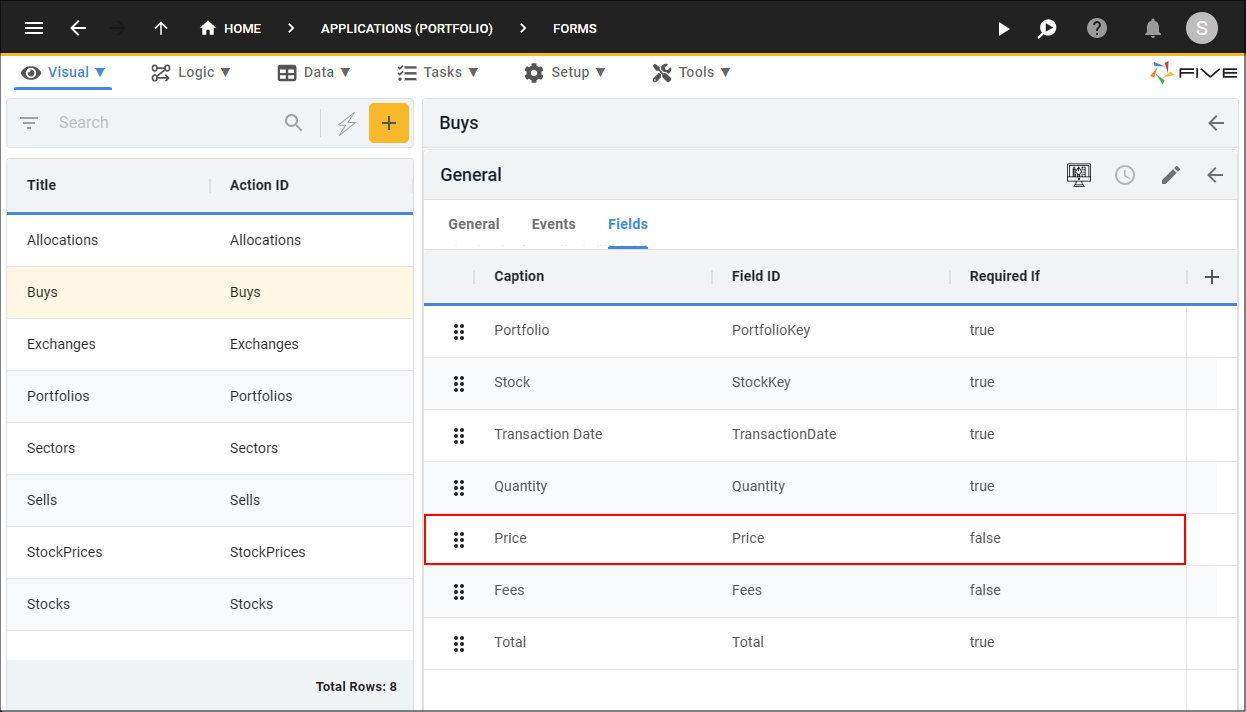
Figure 12 - Price record
8. Click the Events tab.
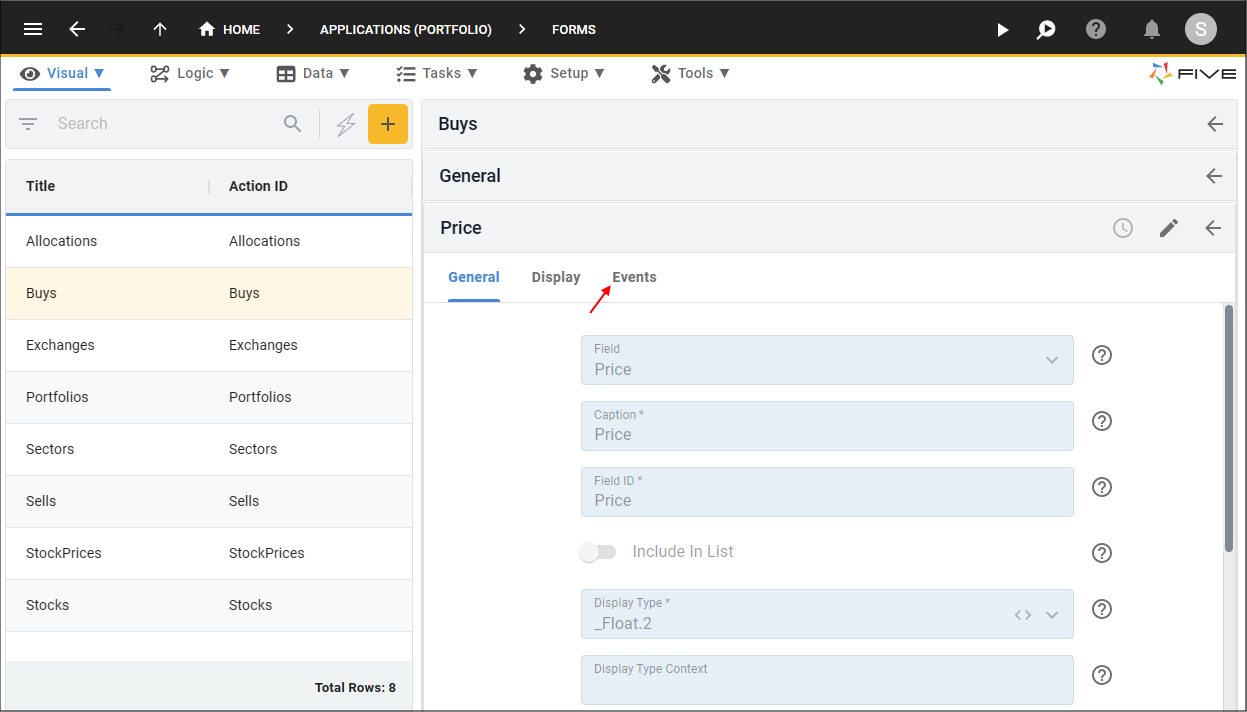
Figure 13 - Events tab
9. Click the lookup icon in the On Validate field and select CheckPrice.
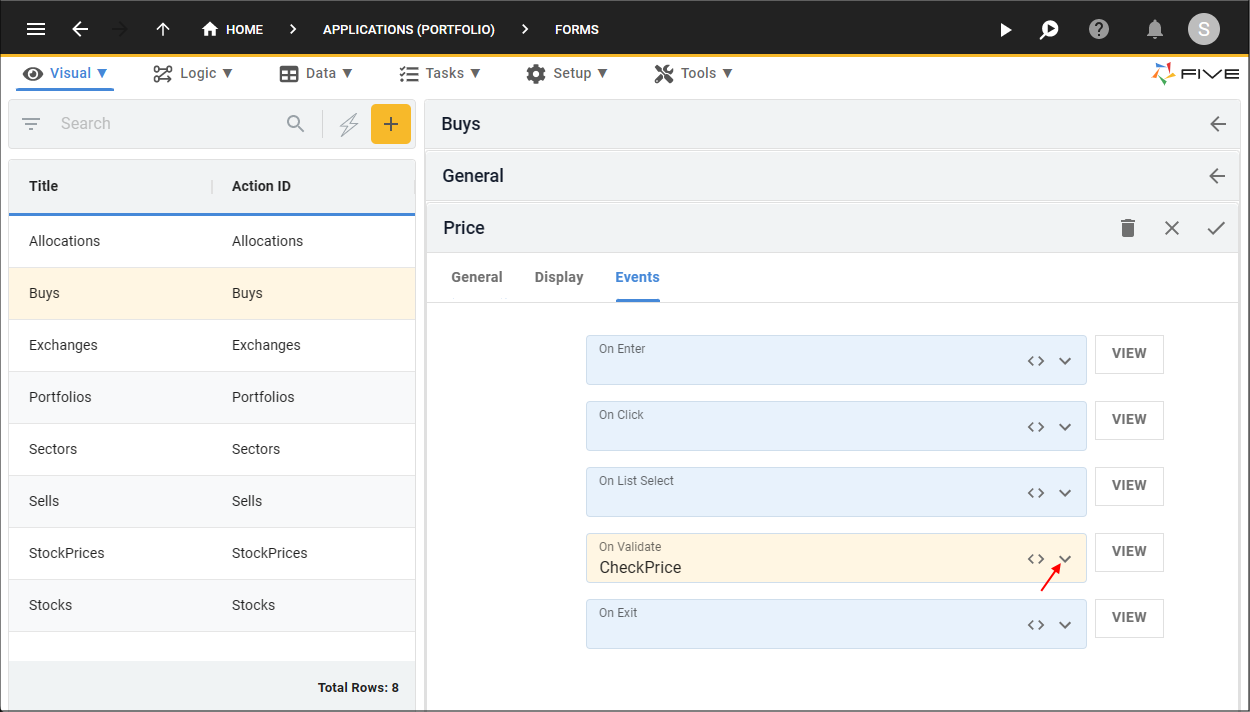
Figure 14 - Attach the CheckPrice function
10. Click the Save button in the form app bar.
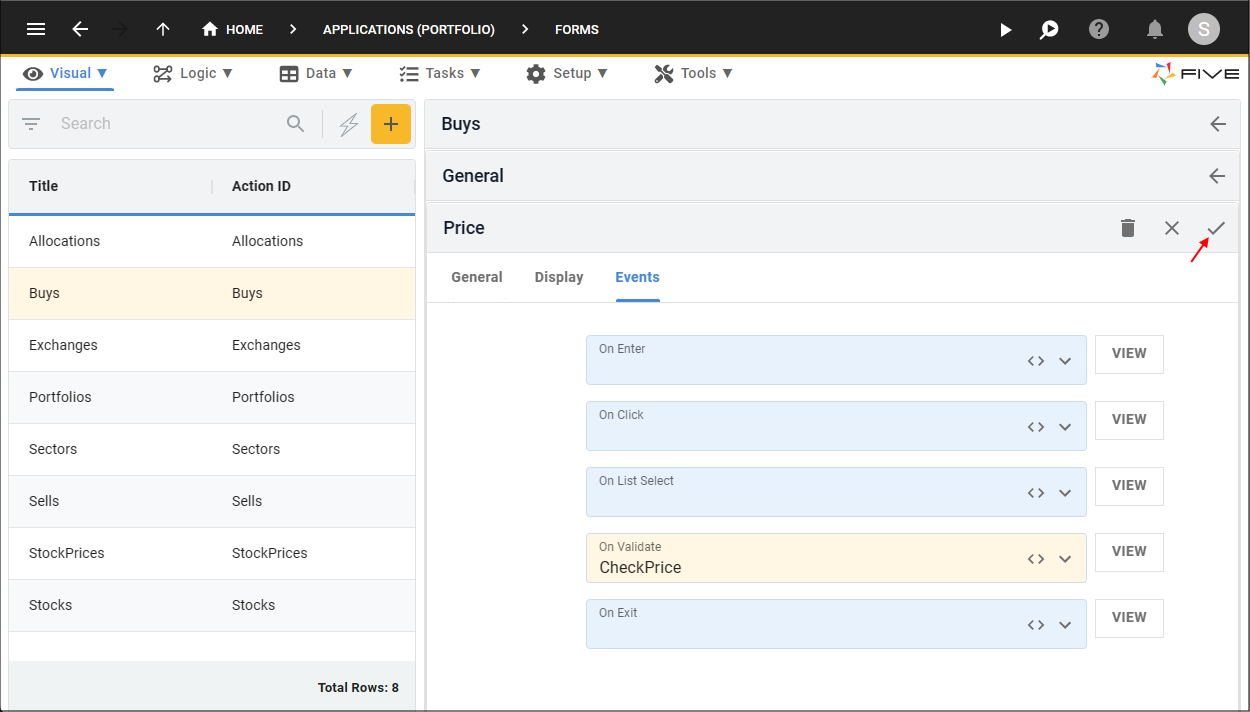
Figure 15 - Save the CheckPrice event
11. Click the Save button in the form app bar above the list.
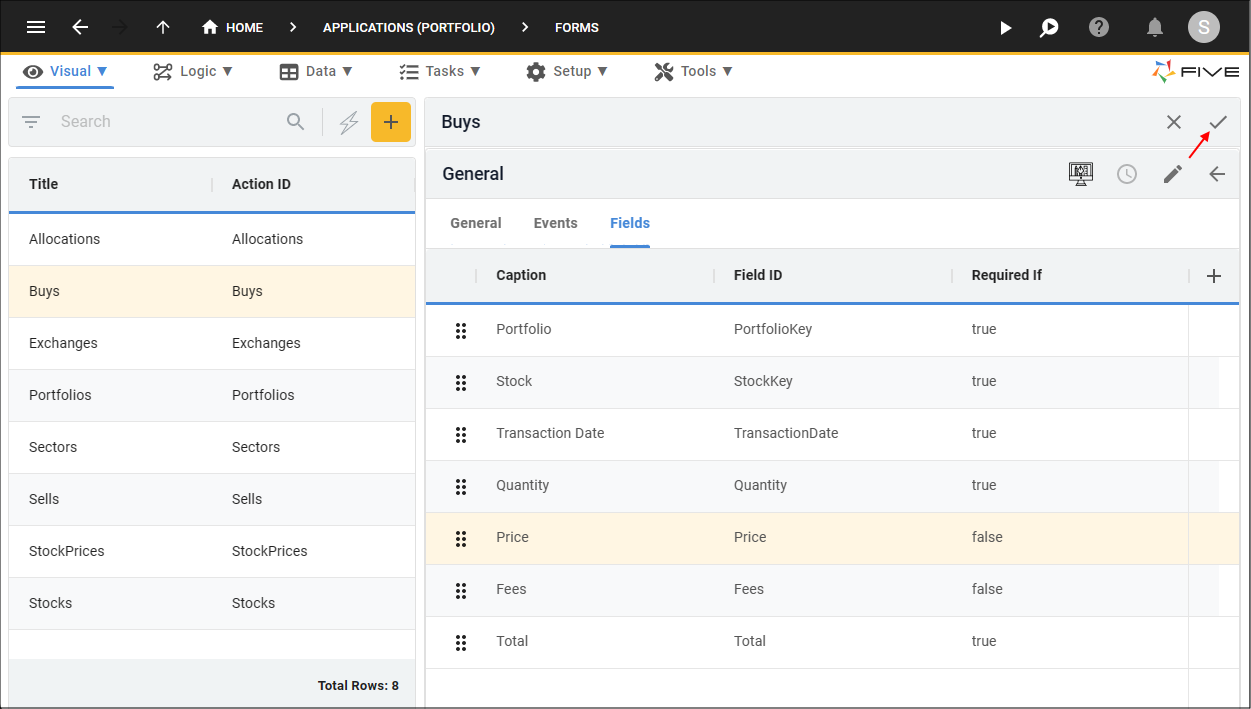
Figure 16 - Save the Buys form
Run the Portfolio Application
1. Click the Run button in Five's toolbar.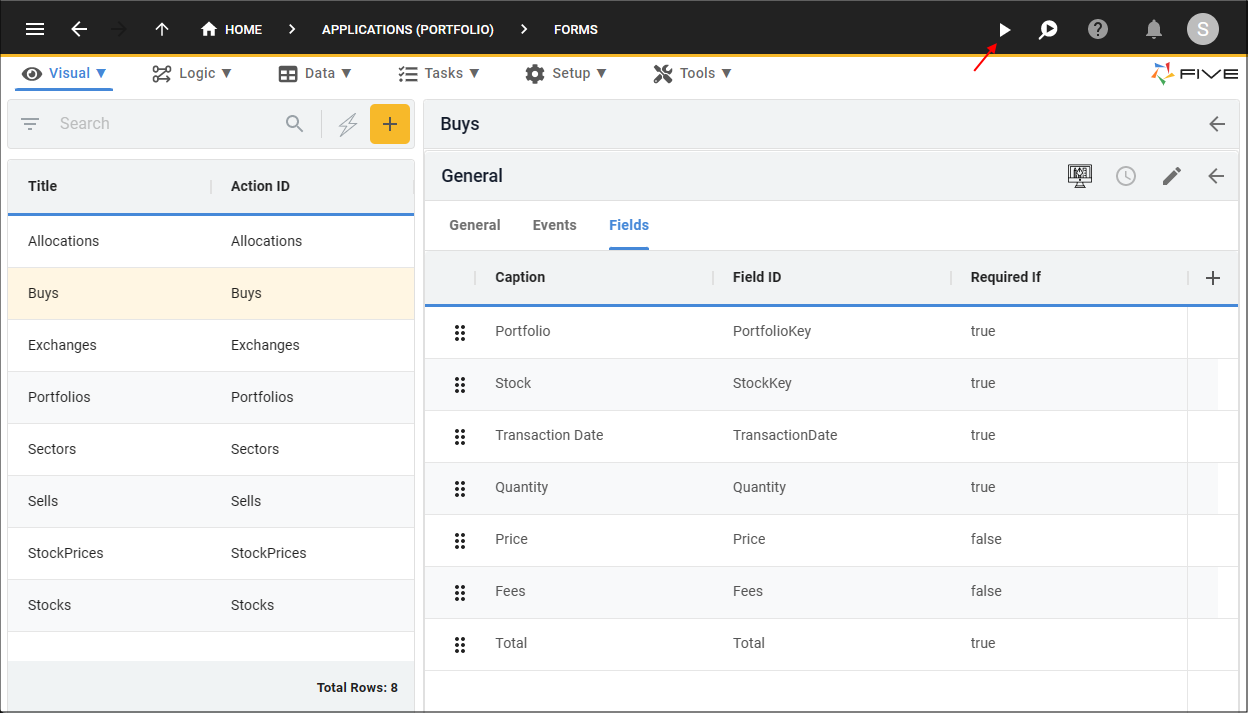
Figure 17 - Run button
2. Select the Growth Portfolio record.
3. Click the Down button in the form app bar.
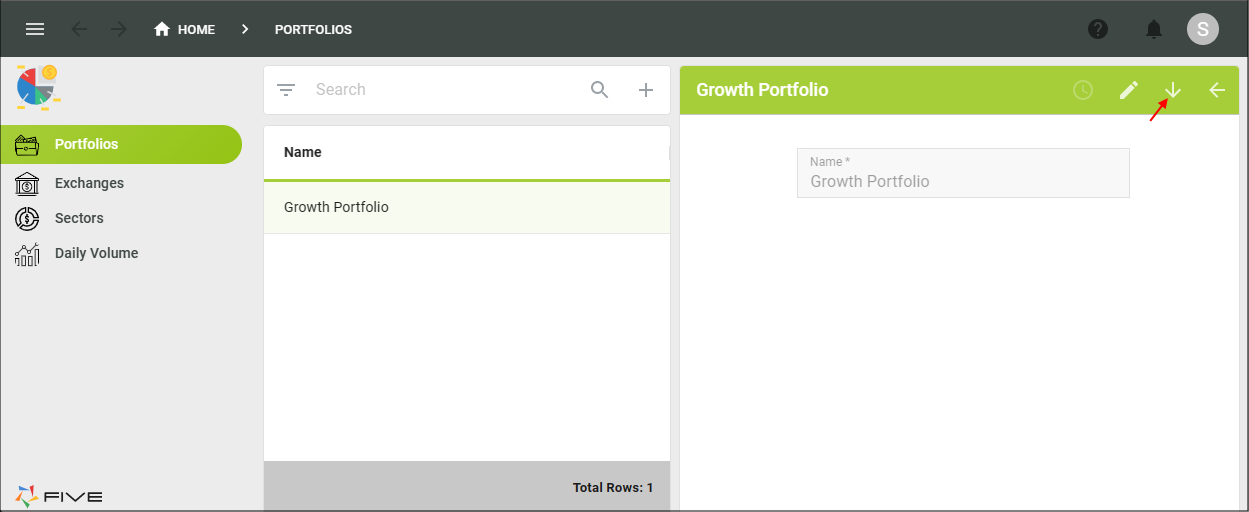
Figure 18 - Down button
Test the CheckPrice Function
1. Select Buys in the menu.2. Click the Add Item button.
3. Click the lookup icon in the Stock field and select AEI.
4. Click the calendar icon in the Transaction Date field.
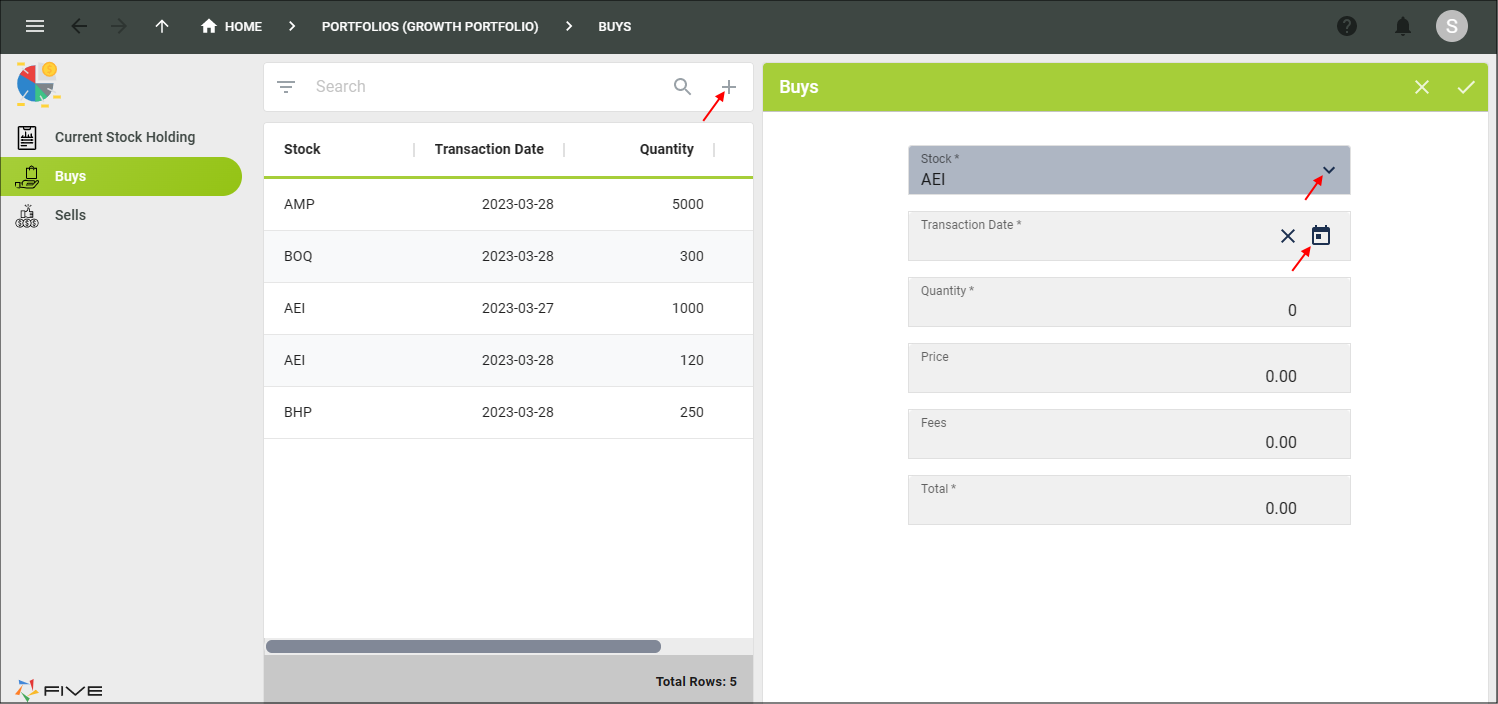
Figure 19 - Add an AEI buy transaction
info
Our selected record will be back dated as we have to work with the data that is currently in the Portfolio application.
The AEI stock record we are going to check had a high price of .30 and a low price of .20 for the date 2019-09-05. You can verify this by looking on the Exchanges or Sectors forms.
The AEI stock record we are going to check had a high price of .30 and a low price of .20 for the date 2019-09-05. You can verify this by looking on the Exchanges or Sectors forms.
5. Select the year 2019.
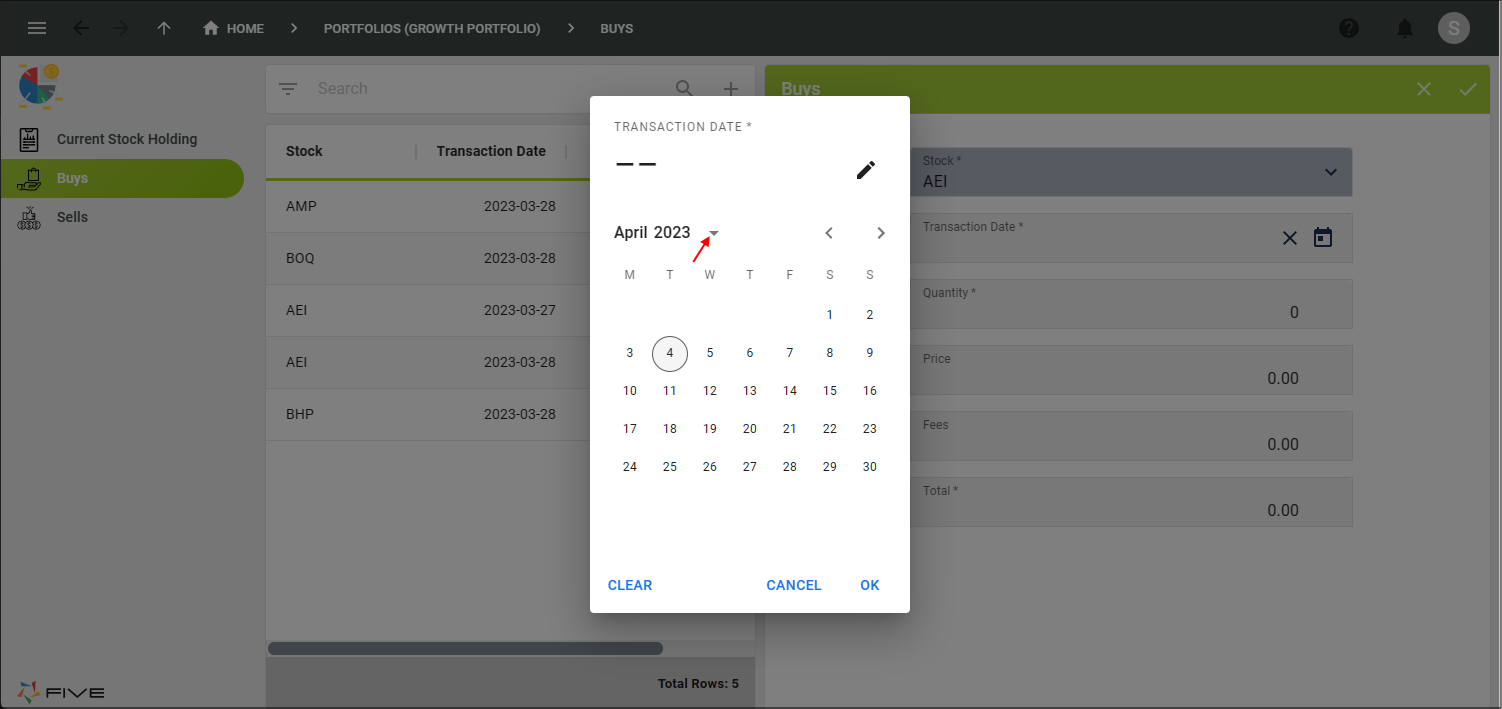
Figure 20 - Select 2019
6. Select the month September.
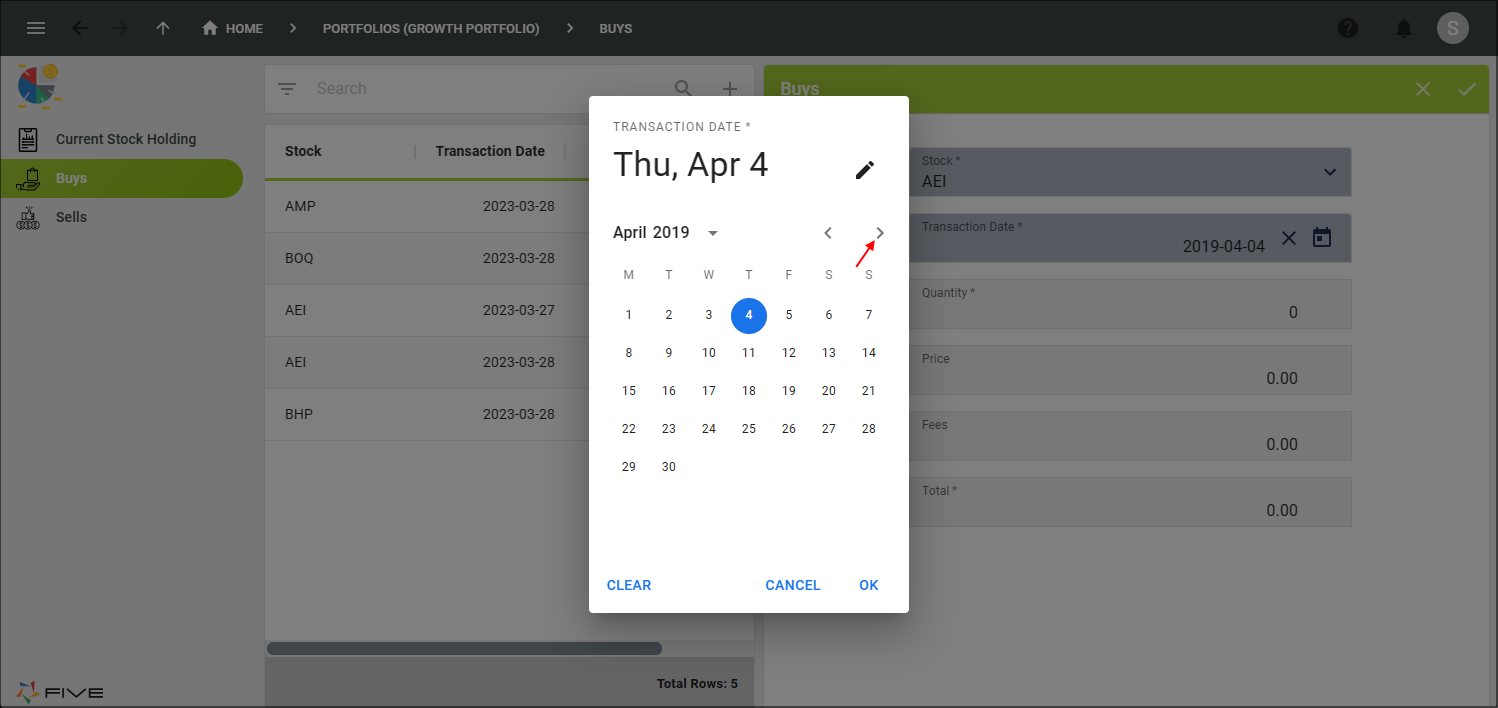
Figure 21 - Select September
7. Select the date 5th.
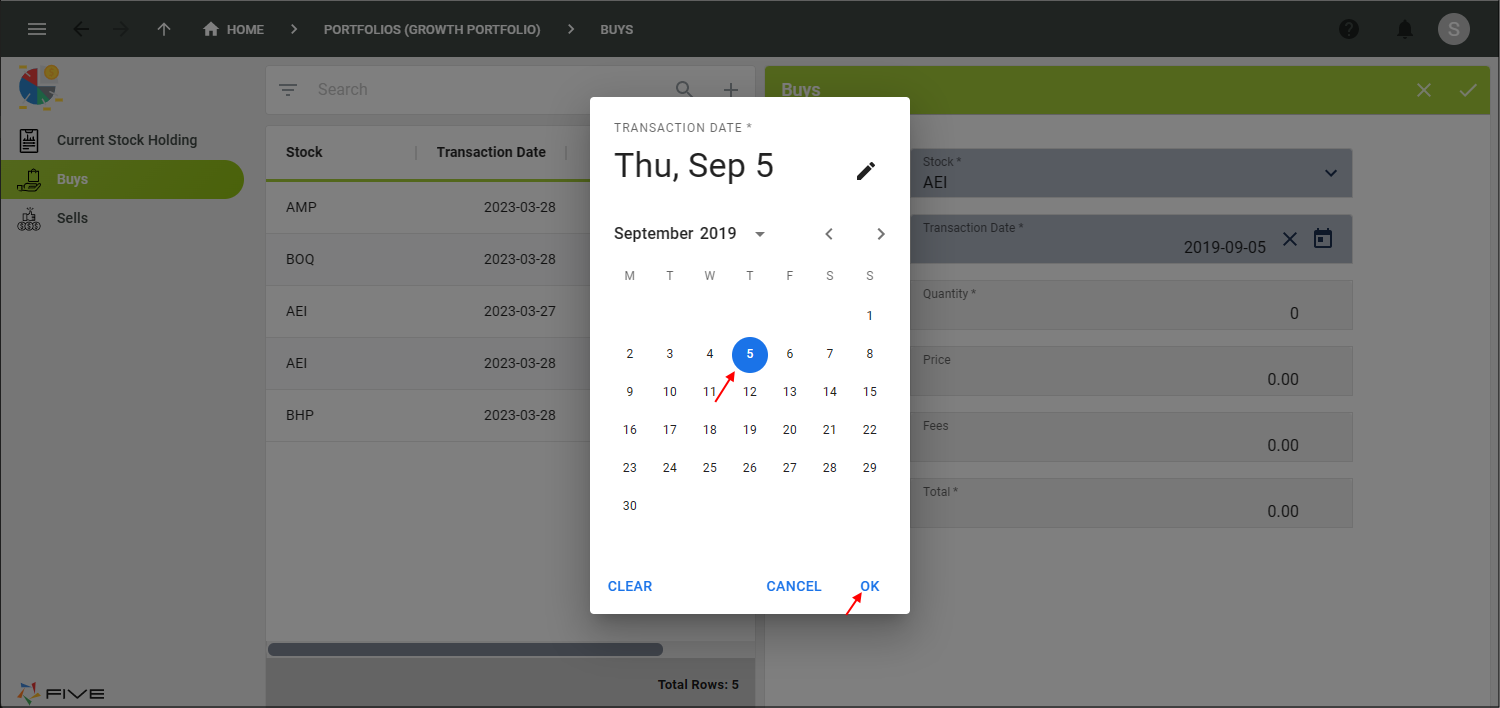
Figure 22 - Select 5th
8. Type 1 in the Quantity field.
9. Type .35 in the Price field, press tab.
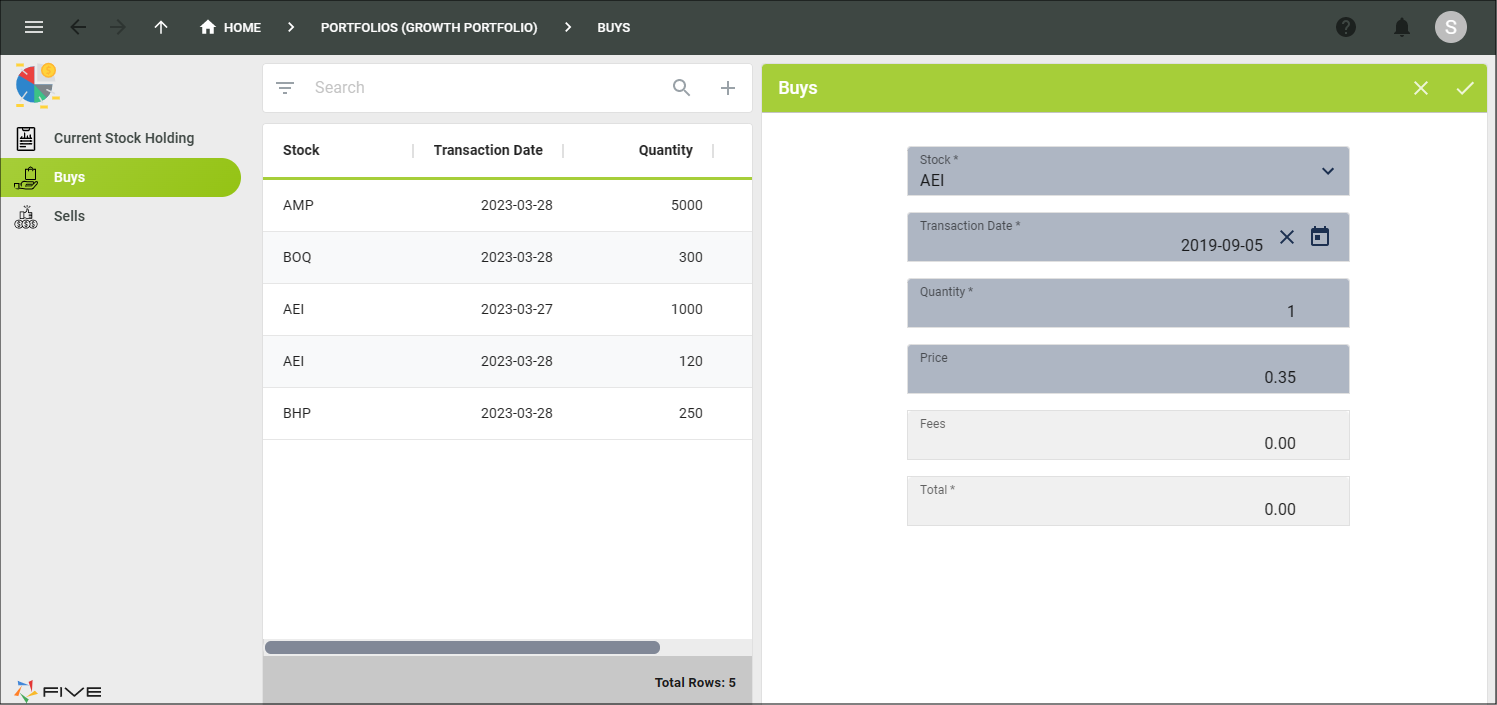
Figure 23 - Above high price
info
An error is returned as we are trying to buy above the high price for the day.
10. Click the OK button.
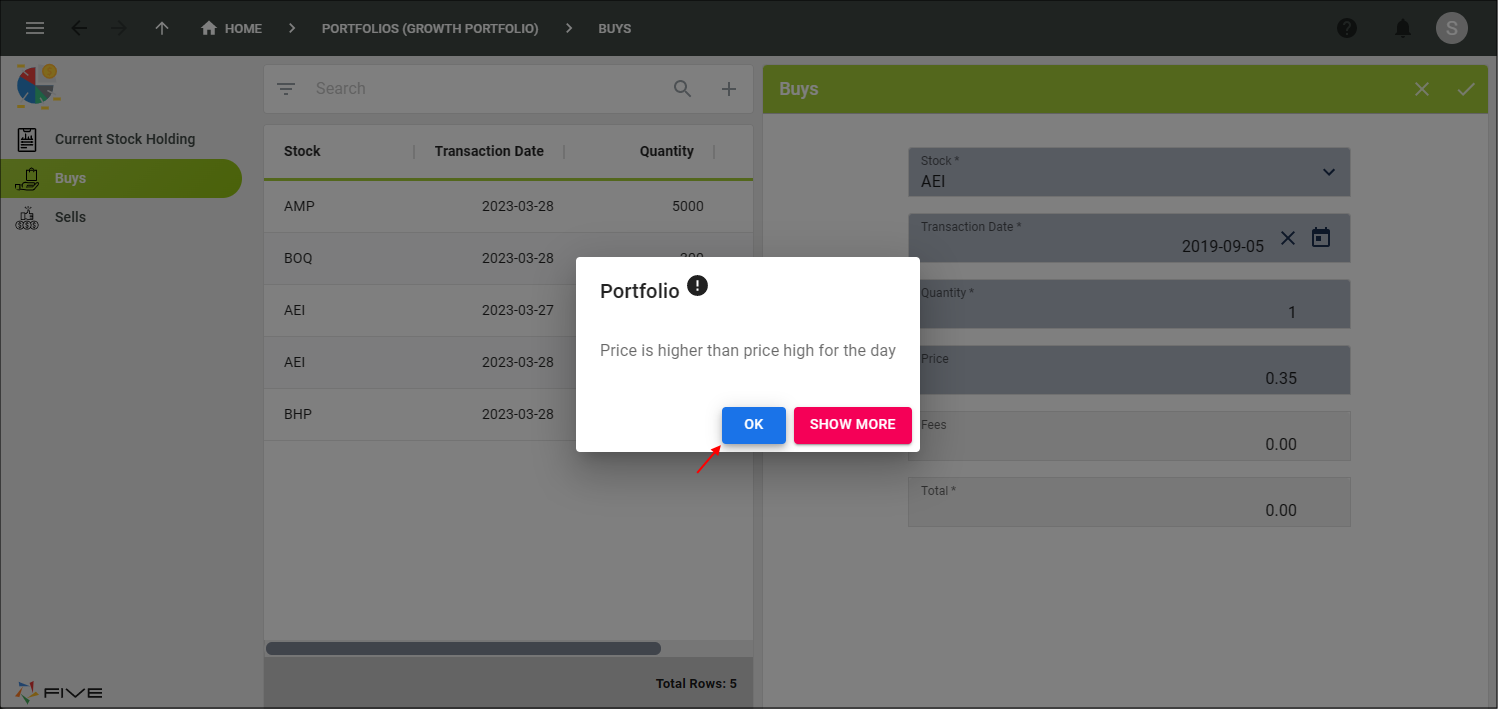
Figure 24 - Above high price for the day error
11. Type .15 in the Price field, press tab.
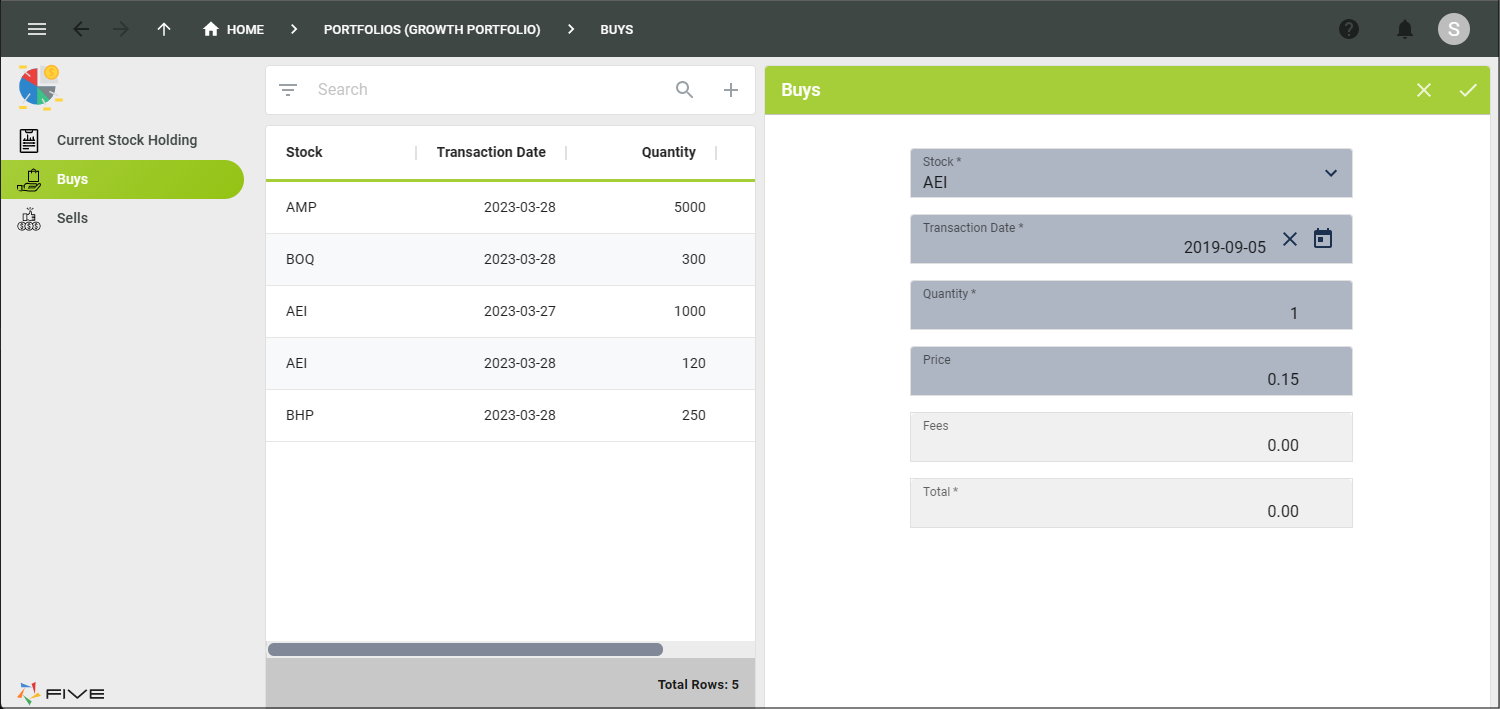
Figure 25 - Below low price
info
An error is returned as we are trying to buy under the low price for the day.
12. Click the OK button.
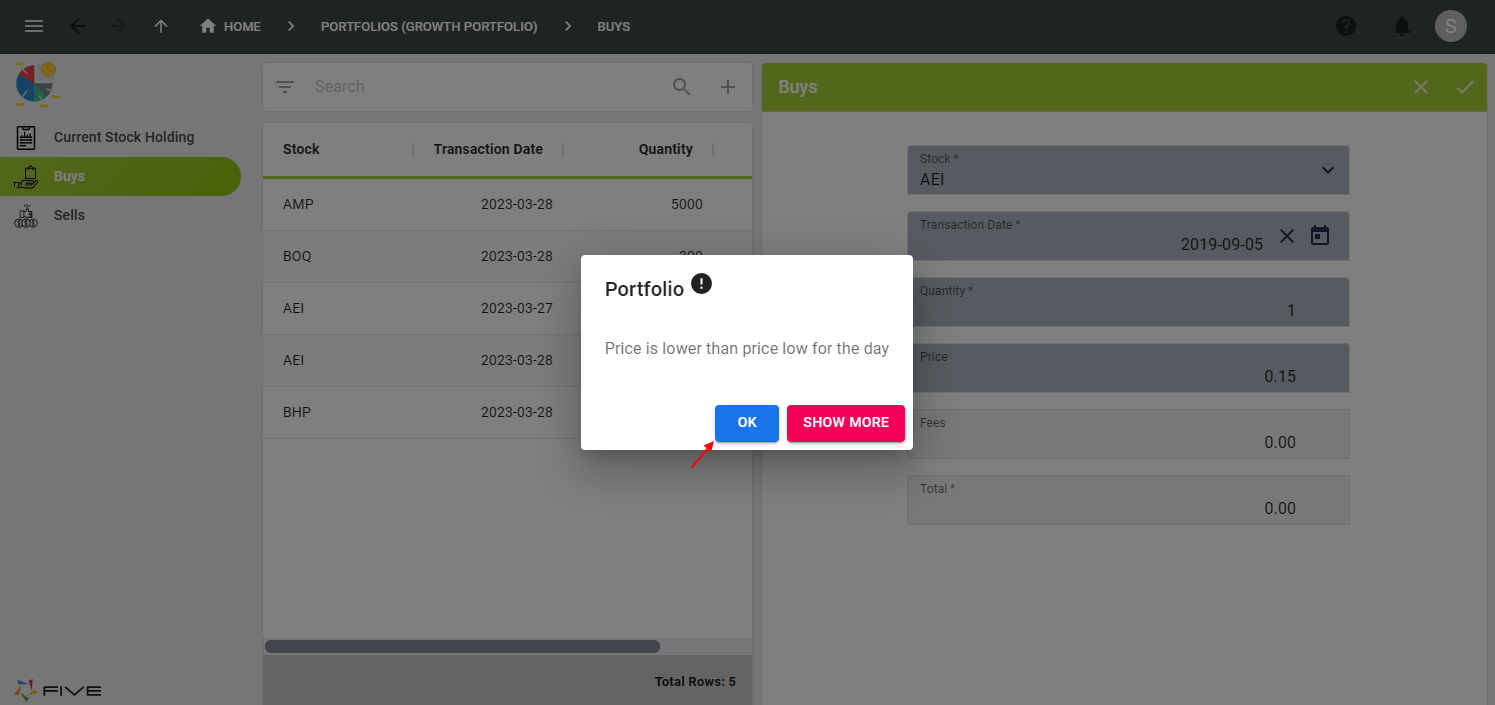
Figure 26 - Below low price for the day error
13. Click the Cancel button in the form app bar.
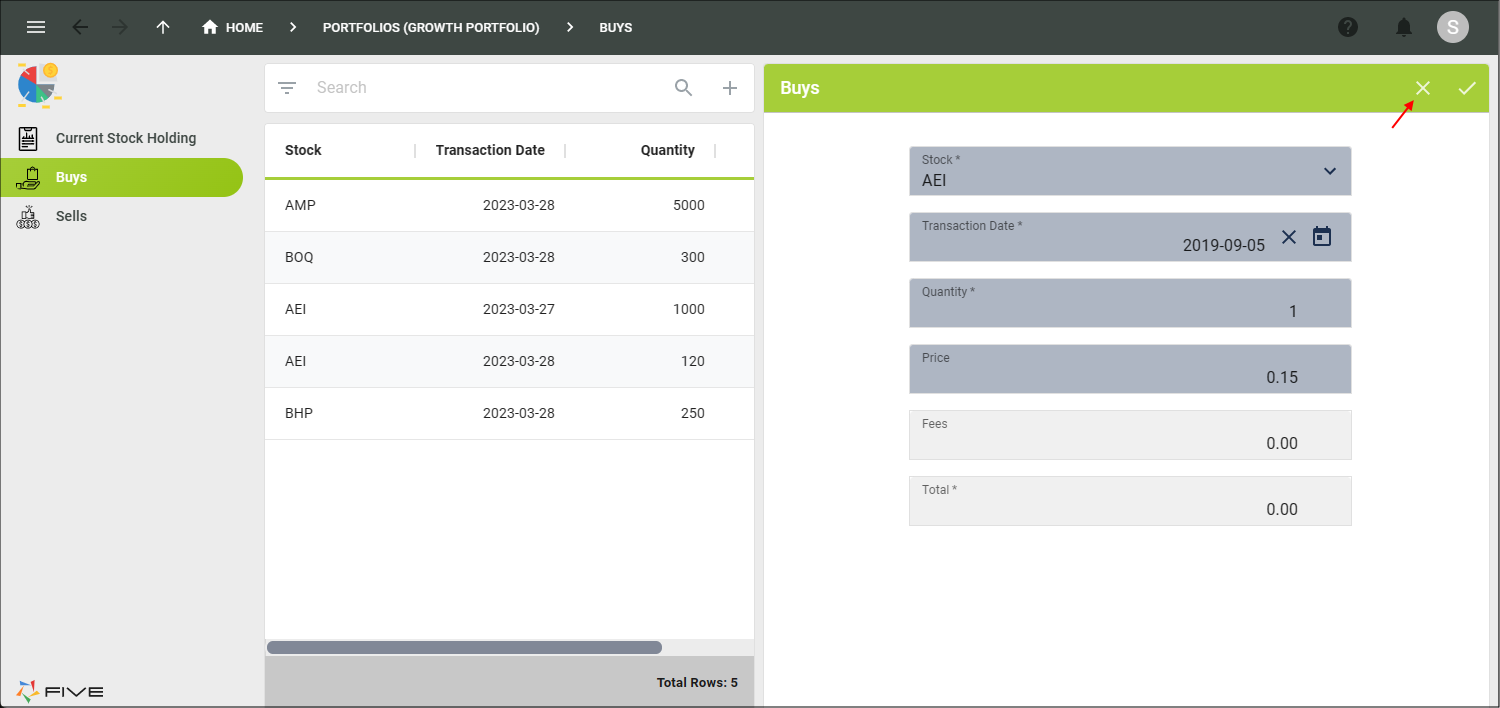
Figure 27 - Cancel button
14. Close the browser tab and return to Five.