Interact with the Backend From the Frontend
Last updated 4/11/2024
This how to guide will demonstrate how to interact with the backend from the frontend to return values from the server to the client. A small application with just one table that has three fields will be used to demonstrate this.
If you want the UI Server Data Transfer application as a reference, click to download the FDF file UIServerDataTF.fdf and you can import this FDF into your Five account.
If you are building the application, you can't have both application ID's called UIServerDataTF.
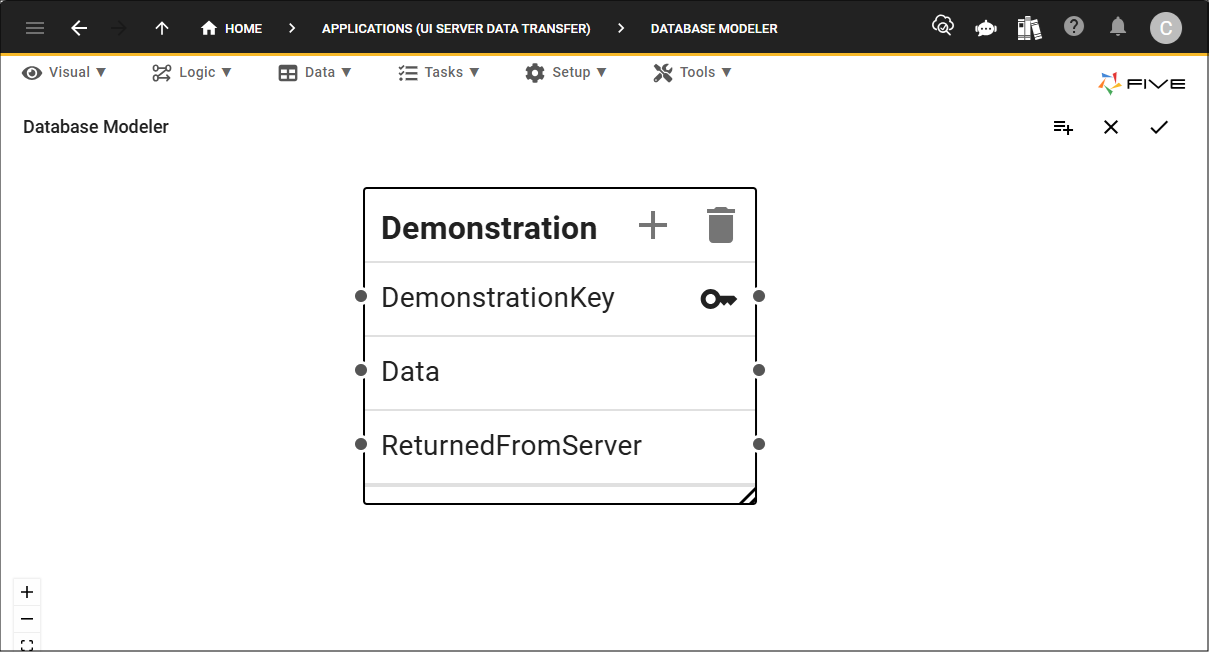
The application has one form that is attached to a menu item. The form has the fields Data and Returned From Server.
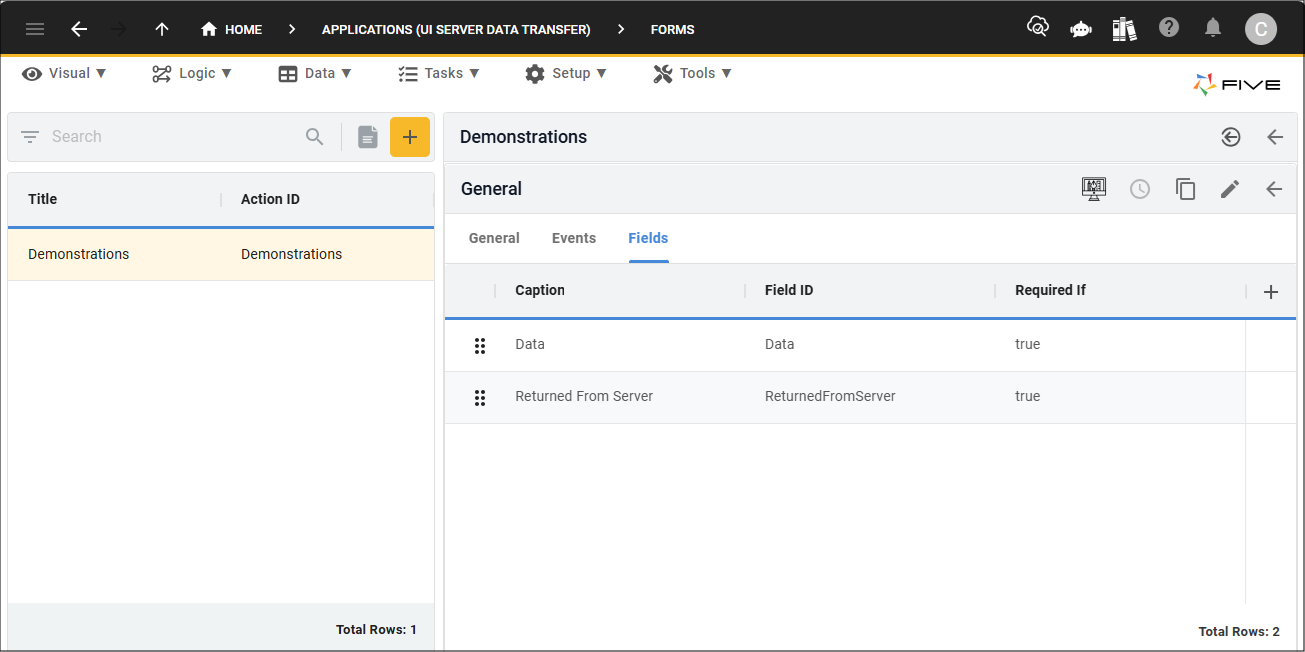
Two functions are in the application. One function will execute in the frontend and call the function that executes on the server.
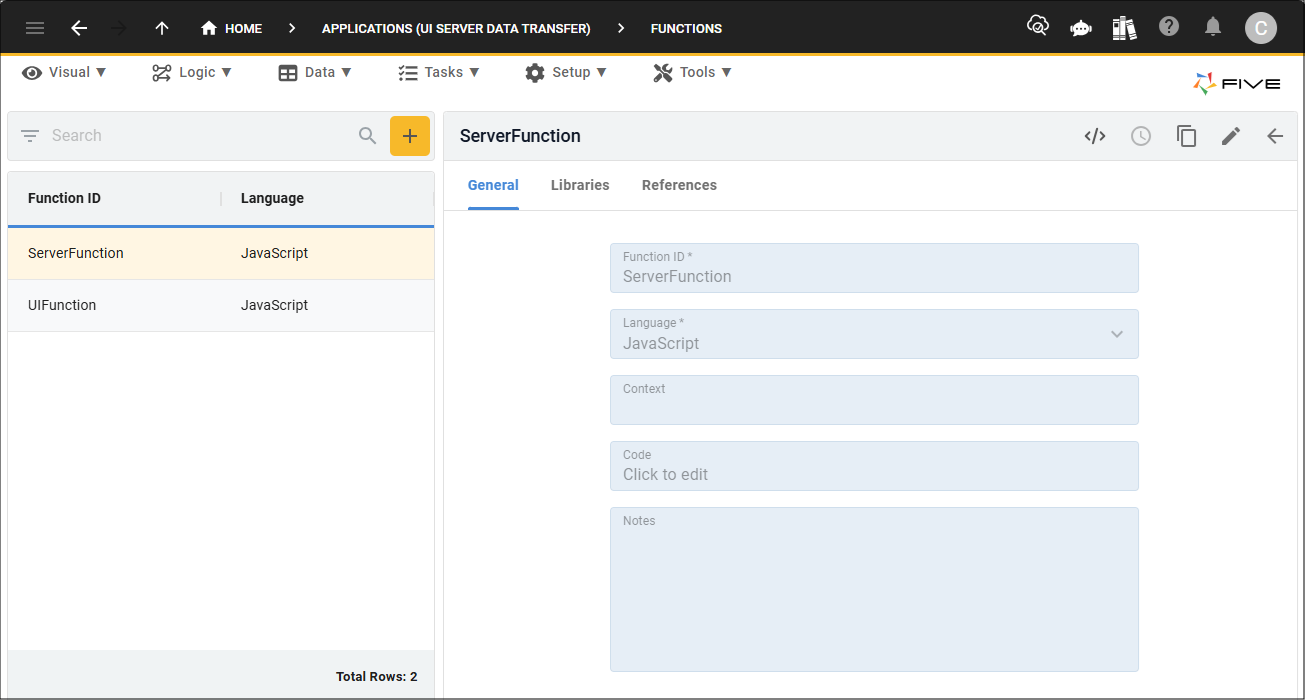
The server function is called
The second parameter in the
A server variable is created called
The
function ServerFunction(five, context, result) {
/////////////////////////////////////////////////////////////////////////////////
// the second parameter to success is an optional message to display to the user
/////////////////////////////////////////////////////////////////////////////////
result = five.success(result, 'Message to UI');
/////////////////////////////////////////////////////////////////////////////////
// create server data to be returned to the front ui
/////////////////////////////////////////////////////////////////////////////////
const serverData = {};
serverData['ValueFromServer'] = 'HELLO_FROM_SERVER : ' + context.DataToServer;
/////////////////////////////////////////////////////////////////////////////////
// set the data onto the result, and tell five to send it as JSON
/////////////////////////////////////////////////////////////////////////////////
result.setData(five.MIMETypeJSON, serverData);
return result;
}
The UI function is called
This function creates the
The callback provided to
The server data that was sent back as JSON and formatted as text is parsed using
function UIFunction(five, context, result) {
// create variables to send to the server
const variables = {};
variables['DataToServer'] = five.field.Data;
const sender = five.sender();
/////////////////////////////////////////////////////////////////////////////////
// this call is async, so we wont be waiting for it to return, instead a callback has
// been passed into the executeFunction to be executed when the data returns
/////////////////////////////////////////////////////////////////////////////////
five.executeFunction('ServerFunction', variables, null, '', '', function (result) {
/////////////////////////////////////////////////////////////////////////////////
// we can check for errors if necessary as follows :
// if (result.serverResponse.errorCode === 'ErrErrorOk') {
// return;
// }
/////////////////////////////////////////////////////////////////////////////////
const data = result.serverResponse.results;
const serverData = JSON.parse(data);
sender.dataManager.setValueByName('ReturnedFromServer', serverData.ValueFromServer);
});
return five.success(result);
}
The UIFunction is attached to the On Exit event on the Data form field.
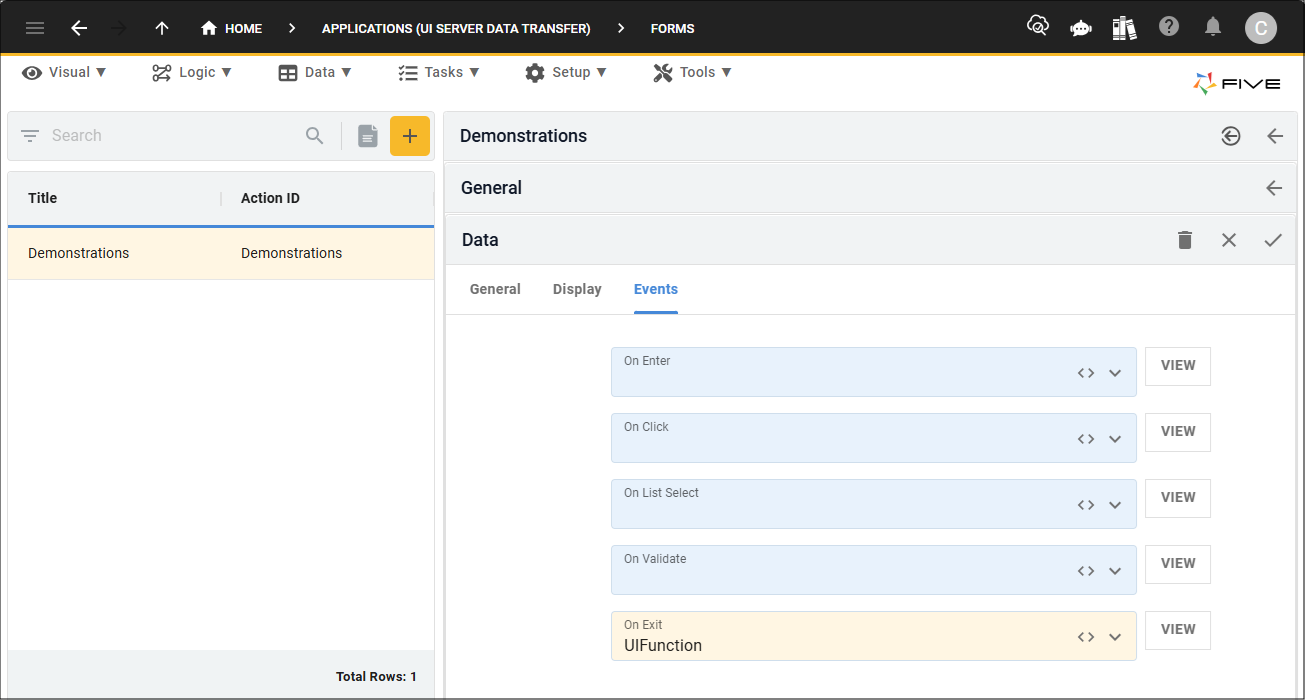
In the application:
- The value is entered into the Data field.
- Upon exiting the Data field, the UIFunction()will execute in the client and call theServerFunction().
- The ServerFunction()will execute and display the message and populate the Returned From Data field with the value from the server.
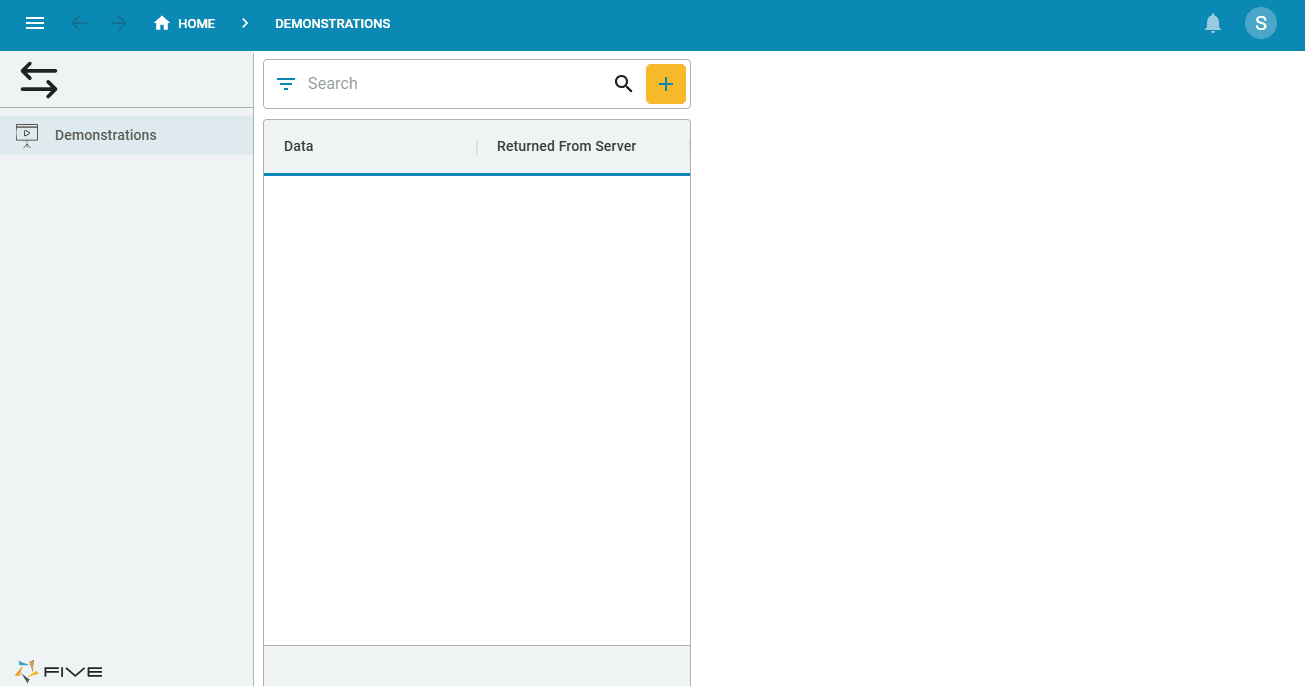