Copy Basic Recipes Card Code
Last updated 17/05/2023
The code provided below is the basic code for the cards in the Recipes application. For more information on styling cards please visit Material UI - Overview.1. Click the Copy button on the code block below.
Basic Cards for Recipe Application
import React from 'react';
import {
FiveInitialize,
Box,
Button,
Card,
CardActions,
CardContent,
Grid,
Typography
} from './FivePluginApi';
FiveInitialize();
const CustomForm = () => {
return (
<Box sx={{ flexGrow: 1 }}>
<Grid container spacing={2}>
<Grid item xs={4} >
<Card sx={{height: "100%",
display: "flex",
flexDirection: "column",}}>
<CardContent>
<Typography gutterBottom variant="h5" component="div">
Marinated Grilled Pork Tenderlion
</Typography>
<Typography variant="body2" color="text.secondary">
This marinated pork loin cooks up nicely on the grill. It tastes best when marinated for 24 hours.
</Typography>
</CardContent>
<CardActions disableSpacing sx={{ mt: "auto" }}>
<Button size="small" onClick={() => {
window.open('https://www.allrecipes.com/recipe/228266/marinated-grilled-pork-tenderloin/', '_parent').focus();
}}>Recipe</Button>
</CardActions>
</Card>
</Grid>
<Grid item xs={4} >
<Card sx={{height: "100%",
display: "flex",
flexDirection: "column",}}>
<CardContent>
<Typography gutterBottom variant="h5" component="div">
Grilled Teriyaki Shrimp and Pineapple Skewers
</Typography>
<Typography variant="body2" color="text.secondary">
These savory-sweet shrimp skewers are easy to make and cook in just a few minutes.
</Typography>
</CardContent>
<CardActions disableSpacing sx={{ mt: "auto" }}>
<Button size="small" onClick={() => {
window.open('https://www.allrecipes.com/recipe/275055/grilled-teriyaki-shrimp-and-pineapple-skewers/', '_parent').focus();
}}>Recipe</Button>
</CardActions>
</Card>
</Grid>
<Grid item xs={4} >
<Card sx={{height: "100%",
display: "flex",
flexDirection: "column",}}>
<CardContent>
<Typography gutterBottom variant="h5" component="div">
Grilled Vegetables with Balsamic Vinegar
</Typography>
<Typography variant="body2" color="text.secondary">
A simple recipe for vegetables that let's their quality and freshness shine through!
</Typography>
</CardContent>
<CardActions disableSpacing sx={{ mt: "auto" }}>
<Button size="small" onClick={() => {
window.open('https://www.allrecipes.com/recipe/222702/grilled-vegetables-with-balsamic-vinegar/', '_parent').focus();
}}>Recipe</Button>
</CardActions>
</Card>
</Grid>
</Grid>
</Box>
);
}
export default CustomForm;
2. Inside your IDE, open the src folder.
3. Open the customForm.tsx file.
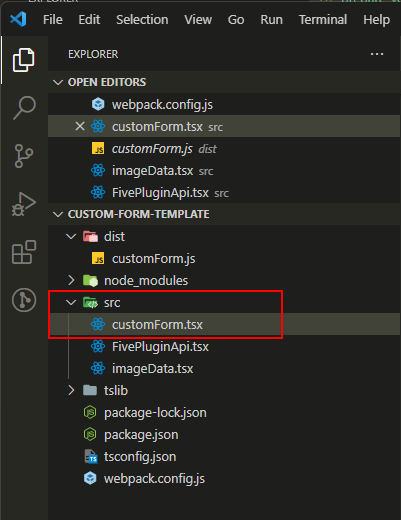
Figure 1 - customForm.tsx
4. Delete the current code in your IDE.
5. Paste the above code into your IDE.
6. Save the code in your IDE.
7. In the terminal run the webjet command again.
node ./node_modules/webpack/bin/webpack.js